How to Add Elements in Array in Java
admin
0 Comments
for loop, how to add elements in array in java using for loop, java array sum, program to find sum of array elements in java, sum of array elements in java solution, sum of array in java using, sum of elements in an array java
In this tutorial, we are going to see two programs for calculating the sum of all the elements of an array in Java. The first program finds the sum of the elements of the specified array. The second program takes the numbers provided by the user and finds the sum of those numbers using an array.
Example 1: Program to Add Elements in Array
class Main { public static void main(String args[]){ int[] arr = {1, 2, 3, 4, 5}; int sum = 0; for( int i : arr) { sum = sum + i; } System.out.println("The sum of the array elements is : "+ sum); } }
Output:
The sum of the array elements is : 15
Example 2: Calculate the sum of the numbers entered by the user
import java.util.Scanner; class Main { public static void main(String args[]){ Scanner scanner = new Scanner(System.in); int[] arr = new int[10]; int sum = 0; System.out.println("Enter the elements of the array: "); for (int i=0; i < 5; i++) { arr[i] = scanner.nextInt(); } for( int i : arr) { sum = sum + i; } System.out.println("The sum of the array elements is : "+ sum); } }
Output:
Enter the elements of the array: 1 2 3 4 5 The sum of the array elements is : 15
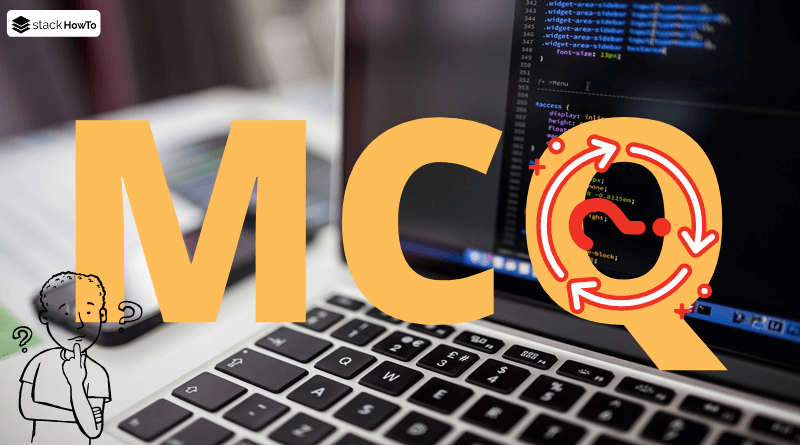
- JFrame Exit on Close Java Swing
- How to Set JFrame in Center of the Screen
- How to Fix the Size of JFrame in Java
- How to Set the Title of a JFrame in Java
- How to Change the Size of a JFrame(window) in Java
- JFrame – Java Swing – Example
- JPanel – Java Swing – Example
- JLabel – Java Swing – Example
- JButton – Java Swing – Example
- JTextField – Java Swing – Example
- JTextArea – Java Swing – Example
- JCheckBox – Java Swing – Example
- JRadioButton – Java Swing – Example
- JComboBox – Java Swing – Example
- JMenu, JMenuBar and JMenuItem – Java Swing – Example
- JDialog – Java Swing – Example
- Dialog boxes – JOptionPane – Java Swing – Example
- JProgressBar – Java Swing – Example
- JPasswordField – Java Swing – Example
- JFileChooser – Java Swing – Example
- JSlider – Java Swing – Example
- JSpinner – Java Swing – Example
- JTree – Java Swing – Example
- JToolBar – Java Swing – Example
- JList – Java Swing – Example
- JToggleButton – Java Swing – Example
- JSeparator – Java Swing – Example
- JColorChooser – Java Swing – Example
- FlowLayout – Java Swing – Example
- GridLayout – Java Swing – Example
- BorderLayout – Java Swing – Example
- BoxLayout – Java Swing – Example
- CardLayout – Java Swing – Example
- GridBagLayout – Java Swing – Example
- JComponent – Java Swing – Example
- GroupLayout – Java Swing – Example
- SpringLayout – Java Swing – Example
- JLayeredPane – Java Swing – Example
- JSplitPane – Java Swing – Example
- ScrollPaneLayout – Java Swing – Example
- Event and Listener – Java Swing – Example
- ActionListener – Java Swing – Example
- MouseListener – Java Swing – Example
- KeyListener – Java Swing – Example
- How to Change Java Icon in JFrame
- How to Change Font Size and Font Style of a JLabel
- How to Count the Clicks on a Button in Java
- How to Get Mouse Position on Click Relative to JFrame
- How to Change Look and Feel of Swing Application
- How to display an image on JFrame in Java Swing
- How to Add an Image to a JPanel in Java Swing
- How to Change Font Color and Font Size of a JTextField in Java Swing
- How to Display Multiple Lines in Tooltip
- How to dynamically filter JTable from textfield in Java
- How to get Value of Selected JRadioButton in Java
- How to get the selected item of a JComboBox in Java
- How to Populate JTable from Database
- How to insert and retrieve an image from MySQL database using Java
- How to Create a Vertical Menu Bar in Java Swing
- How to add real-time date and time in JFrame
- Use Enter key to press JButton instead of mouse click
- How to add text to an image in Java
- How to Clear JTextArea by Clicking JButton
- How to use JFileChooser to display image in a JFrame
- How to Get the State of JCheckBox in Java Swing
- How to link two JComboBox together in Java Swing
- How to Display Multiple Images in a JFrame
- How to draw lines, rectangles, and circles in JFrame
- How to Display a Webpage Inside a Swing Application
- Difference between JTextField and JFormattedTextField in Java
- How to Make JTextField Accept Only Alphabet
- How to Make JTextField Accept Only Numbers
- How To Limit the Number of Characters in JTextField
- How to Capitalize First Letters in a JTextField in Java
- Convert to Uppercase while Writing in JTextField
- How to Add a Listener for JTextField when it Changing
- How to Create Rounded JButton in Java
- How to Disable JButton when JTextField is Empty
- How to Make JButton with Transparent Background
- How to Change JButton Text on Click
- How to Change the Border of a JFrame in Java
- How to Change Font Size in a JButton
- How to Remove Border Around JButton in Java
- How to Remove Border Around Text in JButton
- How to Change Border Color of a JButton in Java Swing
- How to Change Button Color on Click
- How to Change JButton Text Color
- How to Change the Background Color of a JButton
- How to Change the Position of JButton in Java
- How to Add JSpinner to JTable
- How to Update a Row in JTable
- How to Populate Jtable with a Vector
- How to Print a JTable with Image in Header
- How to Delete a Row in JTable using JButton
- How to Add a JComboBox to a JTable Cell
- How to Get Selected Value from JTable in Java
- How to Hide a Column in JTable
- How to Sort JTable Column in Java [2 Methods]
- How to Change Color of Column in JTable
- How to Alternate Row Color of JTable in Java
- How to Change Background Color of JTable Cell on Mouse Click
- How to Count Number of Rows and Columns of a JTable
- How to Add Button in JTable
- How to add JCheckBox in JTable
- How to Add Row Dynamically in JTable Java
- How to Display Image in JTable in Java
- How to Create Multi-Line Header for JTable
- How to Set Column Width in JTable in Java
- How to Increase Row Height in JTable
- How to Remove Jtable Header in Java
- How to Use setBounds() Method in Java
- How to Know Which Button is Clicked in Java Swing
- How to Close a JFrame in Java by a Button
- How to add onclick event to JButton using ActionListener in Java Swing
- How to add checkbox in menuItem of jMenu in Java Swing
- How to create a right-click context menu in Java Swing
- How to Customize JComboBox in Java
- How to Create Hyperlink with JLabel in Java
- How to add an object to a JComboBox in Java
- How to add and remove items in JComboBox in Java
- How to Add Image Icon to JButton in Java Swing
- How to Disable JTextArea and JtextField
- How to Create Multiple Tabs in Java Swing
- How to create a custom cursor in Java
- How to resize an image in Java
- How to Set Background Image in Java Swing
- How to Delete a Selected Row from JTable in Java
- How to Change Background Color of a Jbutton on Mouse Hover
- Detect Left, Middle, and Right Mouse Click – Java
- How to Create Executable JAR File in Java
- Export Data from JTable to Excel in Java
- Java MCQ – Enumerations
- Java MCQ – Data structures (Arrays)
- Java MCQ – Generic types
- Java 8 MCQ Online Test – Part 1
- Java 8 MCQ Online Test – Part 2
- J2EE MCQ with Answers
- Java MCQ – Classes and Objects
- Java MCQ – JDK JRE JVM and JIT
- Java MCQ – Exception Handling – Part 1
- Java MCQ – Exception Handling – Part 2
- Java MCQ – Collections – Part 1
- Java MCQ – Collections – Part 2
- Java MCQ – Collections – Part 3
- Java MCQ – Collections – Part 4
- Java MCQ – Interfaces – Part 1
- Java MCQ – Interfaces – Part 2
- Java MCQ – Multiple Choice Questions and Answers – Data Types and Variables – Part 1
- Java MCQ – Multiple Choice Questions and Answers – Data Types and Variables – Part 2
- How to iterate through a HashMap in Java
- How to get the length or size of an ArrayList in Java
- How to initialize a list with values in Java
- How to Clone or Copy a List in Java
- How to Extract Text Between Parenthesis in Java
- How to remove text between tags using Regex in Java
- How to Get String Between Two Tags in Java
- How to extract email addresses from a string in Java
- How to extract numbers from a string with regex in Java
- How to calculate the average of an ArrayList in Java
- How to find the sum of even numbers in Java
- How to open a file in Java
- How to read the contents of a file into a String in Java
- How to read the first line of a file in Java
- Java Program to Write into a Text File
- How to read a specific line from a text file in Java
- How to fill a 2D array with numbers in Java
- How to add a character to a string in Java
- How to extract numbers from an alphanumeric string in Java
- How to Compare Two ArrayList in Java
- How to check if an element exists in an array in Java
- How to export data to CSV file in Java
- Phone number validation using regular expression (regex) in Java
- How to escape special characters in Java
- How to check operating system in Java
- How to determine the class name of an object in Java
- How to hash a string with MD5 in Java
- How to hash a string with sha256 in Java
- How to send mail in Java using Gmail
- How to Get File Creation Date in Java
- How to create a temporary file in Java
- How to delete a directory if exists in Java
- How to Check if a Folder is Empty in Java
- How to Copy a Directory in Java
- How to check Java version in Windows, Linux, or Mac
- How to get MAC address in Java
- How to extract WAR files in Java
- How to create an XML file in Java
- How to remove XML Node using Java DOM Parser
- How to update node value in XML using Java DOM
- How to change an attribute value in XML using Java DOM
- How to add child node in XML using Java DOM
- How to iterate through an ArrayList in Java
- How to add days to date in java
- Java Program to Check Whether a Date is Valid or Not
- How to check if a key exists in a HashMap in Java
- How to pause a Java program for X seconds
- Mutable and Immutable in Java
- How to Count Number of Elements in a List in Java
- How to run a batch file from Java Program
- How to convert an integer to a string in Java
- How to Declare and Initialize two dimensional Array in Java
- How to generate a random string in Java
- How to get values and keys from HashMap in Java
- How to get the first and last elements from ArrayList in Java
- How to extract a substring from a string in Java
- String Concatenation in Java
- How to search a character in a string in Java
- How to iterate through a list in Java
- How to find an element in a list in Java
- How to make a File Read-Only in Java
- How to convert a file into byte array in Java
- How to change the permissions of a file in Java
- How to get the path of a file in Java
- How to list contents of a directory in Java
- How to move a file from one directory to another in Java
- How to append content to an existing file in Java
- How to create a file in Java
- How to copy a file in Java
- How to check if a file exists in Java
- How to get the size of a file in Java
- How to delete a file in Java
- How to rename a file in Java
- How to create a directory if it does not exist in Java
- How to get the current working directory in Java
- How to Convert Array to ArrayList in Java
- How to Convert ArrayList to Array in Java
- How to Merge two ArrayLists in Java
- How to check if a string is null in Java
- How to merge two arrays in Java
- How to check if a string contains only numbers in Java
- How to check if a character is a letter in Java
- How to remove multiple spaces from a string in Java
- How to Convert a String to a Date in Java
- How to round a number to n decimal places in Java
- How to Read a JSON File with Java
- How to Set the Java Path Environment Variable in Windows 10
- How to Compile and Run your Java Program in Command Line
- Why Java Doesn’t Support Multiple Inheritance
- Write a Java Program to Calculate the Area of Circle
- Write a Java Program to Calculate the Area of Triangle
- Write a Java Program to Calculate the Area of Square
- Java Program to Calculate Area of Rectangle
- Java Program to Print Multiplication Table
- Swapping of Two Numbers in Java
- How to Compare Two Strings in Java
- How to Get Current Date and Time in Java
- Java Program to Reverse a Number
- Write a Java Program to Calculate the Multiplication of Two Matrices
- Write a Java Program to Check Whether an Entered Number is Odd or Even
- Binary Search in Java: Recursive + Iterative
- How to search a particular element in an array in Java
- How to convert a char array to a string in Java
- How to Get the IP Address in Java
- Java Program to Convert Decimal to Binary
- Java Program to Convert Decimal to Octal
- Java Program to Convert Decimal to Hexadecimal
- Java Program to Convert Binary Number to Decimal
- Write a Java Program to Multiply Two Numbers
- How to Convert ASCII Code to String in Java
- How to Get the ASCII Value of a Character in Java
- How to Check If a Year is a Leap Year in Java
- Check if a number is positive or negative in Java
- How to Find the Smallest of 3 Numbers in Java
- Java Program to Find Largest of Three Numbers
- Factorial Program In Java In 2 Different Ways
- How to Reverse a String in Java in 2 different ways
- How to Reverse an Array in Java
- How to Add Two Complex Numbers in Java
- Write a Java Program to Add Two Binary Numbers
- Write a Program to Find the GCD of Two Numbers in Java
- How to Sort a String Alphabetically in Java?
- Java Program to Sort an Array in Ascending and Descending Order
- Java Program to Find the Square Root of a Number
- Java Program to Print Pascal Triangle
- How to Read a File Character by Character in Java
- How to read file line by line in Java
- Write a Program to Copy the Contents of One File to Another File in Java
- Java Program to Count the Number of Lines in a File
- How to Count the Number of Occurrences of a Word in a File in Java
- Java Program to Count the Number of Words in a File
- Java – Count the Number of Occurrences in an Array
- Java – Count the Total Number of Characters in a String
- Java – Count Occurrences of a Char in a String
- Program to Count the Number of Vowels and Consonants in a Given String in Java
- Write a Program to Print Odd Numbers From 1 to N
- Write a Program to Print Even Numbers From 1 to N
- Java Program to Find Quotient and Remainder
- Java Program to find Power of a Number
- Sum of Two Matrix in Java
- How to Add Elements in Array in Java
- Calculate the average using array in Java
- Find the Sum of Two Numbers in Java
- Program to Find Transpose of a Matrix in Java
- How to Print an Array in Java
- How to Fill an Array From Keyboard in Java
- How to Get User Input in Java
- Fibonacci Series Program in Java
- Armstrong Number in Java
- How to Check Prime Number in Java
- How to Print Pyramid Triangle Pattern in Java
- Check if a number is a palindrome in Java
- How to Print Prime Numbers From 1 To 100 In Java
- How to generate random numbers in Java
- Eclipse: Keyboard Shortcuts List
- Split a String in Java
- How to download a file from a URL in Java
- How to read the contents of a PDF file in Java
- How to read a file in Java with BufferedReader
- How to Reverse a String in Java Using Recursion
- How to Convert String to Char in Java
- How to Convert Char to String in Java
- How to Calculate the Number of Days Between Two Dates in Java
- How to override the equals() and hashCode() methods in Java
- How to Sort a HashMap by Key and by Value in Java
- Convert Hashmap to List in Java
- Difference between instantiating, declaring, and initializing
- Anonymous Classes in Java
- How to convert Array to List in Java
- How to convert InputStream to OutputStream in Java
- How to split a string in java
- What is a Servlet?
- What Is JUnit Testing?
- How to Merge Two Arrays in Java
- How to initialize an array in Java?
- Comparator and Comparable in Java with example
- What does immutable mean in Java
- How to generate a password with Java
- Difference between StringBuffer and StringBuilder in Java
- How to Shuffle or Randomize a list in Java
- Difference between PrintStream and PrintWriter in Java
- Java 8 Stream Tutorial
- What is Reflection in Java?
- How to randomly select an item from a list in Java
- How to filter a list in Java
- How to reverse a list in Java
- How to iterate a list in reverse order in Java
- How to convert Stream to a Map in Java 8
- How to convert int to string in Java
- Difference between checked and unchecked exception in Java
- Difference between InputStream and OutputStream in Java
- The try-with-resources Statement in Java
- How to convert String to int in Java
- How to find the largest and smallest element in a list in Java
- How to compare two objects in Java
- Autoboxing and Autounboxing in Java
- How to get the index of an element in a list in Java
- Difference between == and .equals()
- How to determine the first day of the week in Java
- How to calculate a number of days between two dates in Java
- How to get the number of days in a particular month of a particular year in Java
- How to get the week of the year for the given date in Java
- How to get a day of the week by passing specific date and time in Java
- How to get the week number from a date in Java
- How to convert InputStream object to String in Java
- How To Convert String To Double In Java
- How To Join List String With Commas In Java
- How to sort items in a stream with Stream.sorted()
- How to Sort an Array in Java
- Java MCQ – Multiple Choice Questions and Answers – Array – Part 1
- Java MCQ – Multiple Choice Questions and Answers – Array – Part 2
- Java MCQ – Multiple Choice Questions and Answers – Strings – Part 1
- Java MCQ – Multiple Choice Questions and Answers – Strings – Part 2
- Java MCQ – Multiple Choice Questions and Answers – Strings – Part 3
- Java MCQ – Multiple Choice Questions and Answers – Strings – Part 4
- Java MCQ – Multiple Choice Questions and Answers – OOPs