JPanel – Java Swing – Example
In this tutorial, we are going to see an example of JPanel in Java Swing. JPanel is part of the Java Swing package, is a container that can store a group of components. The main task of JPanel is to organize the components, various layouts can be defined in JPanel that offer better organization of components, but it does not have a title bar like JFrame.
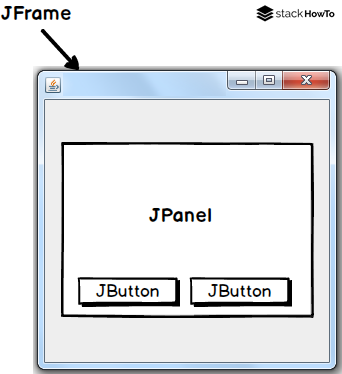
JPanel Constructors:
JPanel() | It is used to create a new JPanel. |
JPanel(LayoutManager l) | Create a new JPanel with the specified layoutManager. |
JPanel(boolean isDoubleBuffered) | Creates a new JPanel with a specified buffering strategy. |
JPanel(LayoutManager l, boolean isDoubleBuffered) | Creates a new JPanel with the specified layoutManager and a specified buffering strategy. |
Example of JPanel in Java Swing:
import java.awt.*; import javax.swing.*; public class JPanelTest { JPanelTest() { //Create the Jframe JFrame f = new JFrame("Welcome To StackHowTo!"); //Create the JPanel JPanel panel = new JPanel(); //Specify the position and size of the JPanel panel.setBounds(40,50,150,150); //Specify the background color of the JPanel panel.setBackground(Color.lightGray); //Create button 1 JButton btn1 = new JButton("Button 1"); //Specify button position and size btn1.setBounds(50,100,80,30); //Specify the background color of the button btn1.setBackground(Color.WHITE); //Create button 2 JButton btn2 = new JButton("Button 2"); btn2.setBounds(100,100,80,30); btn2.setBackground(Color.RED); //Add the two buttons to the JPanel panel.add(btn1); panel.add(btn2); //Add JPanel to JFrame f.add(panel); f.setSize(350,350); f.setLayout(null); f.setVisible(true); } public static void main(String args[]) { new JPanelTest(); } }
Output:
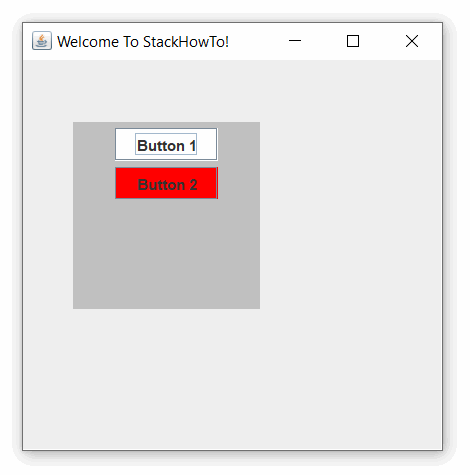