How to create a right-click context menu in Java Swing
JPopupMenu is a class of javax.swing package. It is an implementation of a popup menu. JPopupMenu generates a small window that appears and displays a series of choices. JPopupMenu can be used to generate a small window at any position in a container.
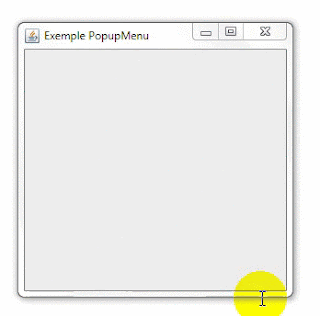
Constructors of JPopupMenu class:
- JPopupMenu(): creates a contextual menu with an empty name
- JPopupMenu(String name): creates a popup menu with the specified title.
Commonly used methods:
- add(JMenuItem menuItem): add menuItem to the context menu.
- add(String s): add a string to the contextual menu.
- getLabel(): get the label of the context menu.
- isVisible(): return whether the JPopup menu is visible or not.
- setLabel(String s): sets the label of the context menu.
- setLocation(int x, int y): sets the location of the context menu to the given coordinates
- setPopupSize(int width, int height): set the size of the popup.
- setVisible(boolean b): set the visibility of the contextual menu, visible if true is passed as argument or vice versa.
- show(Component c, int x, int y): displays the context menu at position x, y in the component c.
Java Program to create a right-click context menu:
import javax.swing.*; import java.awt.event.*; class MyJPopupMenu { MyJPopupMenu() { final JFrame frame = new JFrame("PopupMenu example"); final JPopupMenu menu = new JPopupMenu("Menu"); JMenuItem open = new JMenuItem("Open"); JMenuItem cut = new JMenuItem("Cut"); JMenuItem copy = new JMenuItem("Copy"); JMenuItem paste = new JMenuItem("Paste"); menu.add(open); menu.add(cut); menu.add(copy); menu.add(paste); frame.addMouseListener(new MouseAdapter() { public void mouseClicked(MouseEvent e) { //right mouse click event if (SwingUtilities.isRightMouseButton(e) && e.getClickCount() == 1){ menu.show(frame , e.getX(), e.getY()); } } }); frame.add(menu); frame.setSize(300,300); frame.setLayout(null); frame.setVisible(true); } public static void main(String args[]) { new MyJPopupMenu(); } }
Output:
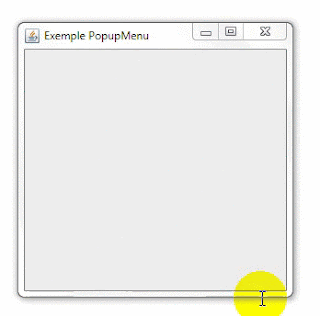