How to Add a JComboBox to a JTable Cell
In this tutorial, we are going to see how to add a JComboBox to a JTable cell. JTable is a flexible Swing component that is very well suited to display data in a tabular format. To add a JComboBox to a JTable cell we will redefine the getCellEditor(…) method of JTable. getCellEditor() method returns the active cell editor, which is null if the table is not being edited.
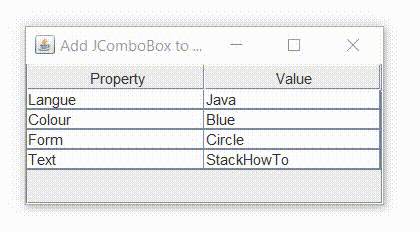
Java Program to Add a JComboBox to a JTable Cell:
import java.awt.*; import java.util.List; import java.util.ArrayList; import javax.swing.*; import javax.swing.table.*; public class ComboBoxJTable extends JPanel { List<String[]> editData = new ArrayList<String[]>(3); public ComboBoxJTable() { setLayout(new BorderLayout()); // data to add to JTable cells editData.add(new String[]{ "Java", "PHP", "Python" }); editData.add(new String[]{ "Red", "Green", "Blue" }); editData.add(new String[]{ "Triangle", "Circle", "Square" }); // create JTable with default data Object[][] data = { {"Langue", "Java"}, {"Colour", "Blue"}, {"Form", "Circle"}, {"Text", "StackHowTo"} }; String[] columns = {"Property", "Value"}; DefaultTableModel model = new DefaultTableModel(data, columns); JTable table = new JTable(model) { // determine which editor to use by JTable public TableCellEditor getCellEditor(int row, int column) { int col = convertColumnIndexToModel(column); if (col == 1 && row < 3) { JComboBox<String> cb = new JComboBox<String>(editData.get(row)); return new DefaultCellEditor(cb); } else return super.getCellEditor(row, column); } }; JScrollPane scroll = new JScrollPane(table); add(scroll); } private static void displayUI() { JFrame f = new JFrame("Add JComboBox to JTable"); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.add(new ComboBoxJTable()); f.setSize(300, 150); f.setLocationByPlatform(true); f.setVisible(true); } public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { displayUI(); } }); } }
Output:
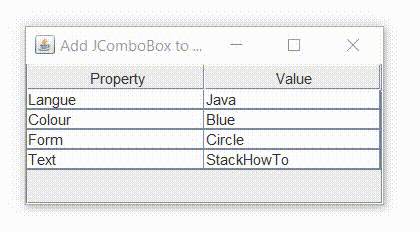