CardLayout – Java Swing – Example
In this tutorial, we are going to see an example of CardLayout in Java Swing. CardLayout class manages components in such a way that only one component is visible at a time. It treats each component as a card, which is why it is called CardLayout.
The constructors of CardLayout class are:
CardLayout() | Arrange the components as a card without horizontal and vertical space. |
CardLayout(int h, int v) | Arrange the components as a card with the given horizontal and vertical space. |
Commonly used methods of CardLayout are the following:
- public void next(Container parent): is used to return to the next card in the given container.
- public void previous(Container parent): is used to return to the previous card of the given container.
- public void first(Container parent): is used to return to the first card of the given container.
- public void last(Container parent): is used to return to the last card of the given container.
- public void show(Container parent, String name): is used to return to the specified card with the given name.
Example of CardLayout in Java Swing
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class MyCardLayout extends JFrame implements ActionListener { CardLayout card; Container c; MyCardLayout() { c = getContentPane(); //create a CardLayout object with 30 hor spaces and 20 ver spaces card = new CardLayout(30,20); c.setLayout(card); JButton btn1 = new JButton("Welcome"); JButton btn2 = new JButton("To"); JButton btn3 = new JButton("StackHowTo"); btn1.addActionListener(this); btn2.addActionListener(this); btn3.addActionListener(this); c.add("a",btn1);c.add("b",btn2);c.add("c",btn3); } public void actionPerformed(ActionEvent e) { card.next(c); } public static void main(String[] args) { MyCardLayout frame = new MyCardLayout(); frame.setSize(300,300); frame.setVisible(true); frame.setDefaultCloseOperation(EXIT_ON_CLOSE); } }
Output:
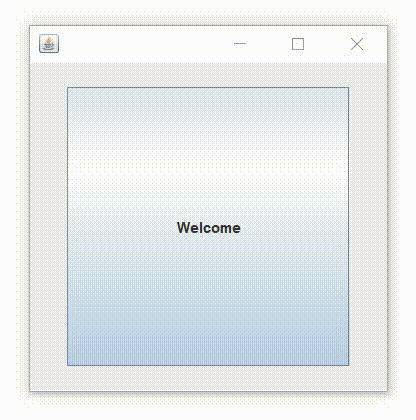