Difference between JTextField and JFormattedTextField in Java
In this tutorial, we are going to see difference between JTextField and JFormattedTextField in Java. JTextField can be used for plain text while a JFormattedTextField is a class that can extend JTextField and it can be used to define any format for the text it contains phone numbers, email addresses, dates, etc.
JTextField
- JTextField is one of the most important components that allow the user to enter a text value in a single line.
- JTextField can generate ActionListener interface when we try to enter a value into the text field and it can generate CaretListener interface every time the cursor changes its position.
- JTextField can also generate MouseListener and KeyListener interfaces.
Example :
import java.awt.*; import javax.swing.*; public class JTextFieldExample extends JFrame { public JTextFieldExample() { setTitle("JTextField Example"); setLayout(new FlowLayout()); JTextField text = new JTextField(20); add(text); setSize(350,80); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLocationRelativeTo(null); setVisible(true); } public static void main(String args[]) { new JTextFieldExample(); } }
Output:
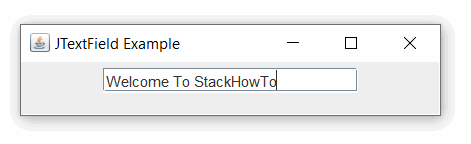
JFormattedTextField
JFormattedTextField class is a subclass of JTextField class.
- JFormattedTextField is like JTextField, except that it checks the validity of the characters and can be associated with a Formatter that specifies the characters that the user can enter.
- JFormattedTextField is a subclass of Format class for creating a formatted text field. We can create a Formatter, customize it if necessary. We can call the constructor JFormattedTextField(Format format) which takes an argument of type Format.
Example :
import java.awt.*; import javax.swing.*; import javax.swing.text.*; public class JFormattedTextFieldExample extends JFrame { JFormattedTextField text; public JFormattedTextFieldExample() { setTitle("JTextField Example"); setLayout(new FlowLayout()); // Format a phone number try { MaskFormatter formatter = new MaskFormatter("##-##-##-##-##"); formatter.setPlaceholderCharacter('#'); text = new JFormattedTextField(formatter); text.setColumns(20); } catch(Exception e) { e.printStackTrace(); } add(text); setSize(350,80); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLocationRelativeTo(null); setVisible(true); } public static void main(String args[]) { new JFormattedTextFieldExample(); } }
Output:
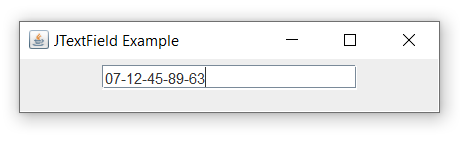