How to Change Background Color of a Jbutton on Mouse Hover
In this tutorial, we are going to see how to change the background color of a Jbutton on mouse hover. We can implement MouseListener interface to manage mouse events. The MouseEvent is triggered when we can press, release or click a mouse button on the source object or position the mouse pointer at the input and or output of the source object. We can detect a mouse event when the mouse moves over a component such as a button using mouseEntered() and mouseExited() method of MouseAdapter class or MouseListener interface.
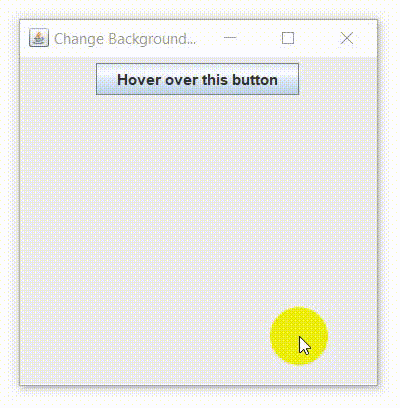
How to Change the Background Color of a Jbutton on Mouse Hover
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class DetectMouseMove extends JFrame { private JButton button; public DetectMouseMove() { setTitle("Change Background Color on Hover"); setLayout(new FlowLayout()); button = new JButton("Hover over this button"); button.setOpaque(true); add(button); button.addMouseListener(new MouseAdapter() { public void mouseEntered(MouseEvent evt) { button.setBackground(Color.ORANGE); } public void mouseExited(MouseEvent evt) { button.setBackground(null); } }); setSize(300, 300); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLocationRelativeTo(null); setVisible(true); } public static void main(String[] args) { new DetectMouseMove(); } }
Output:
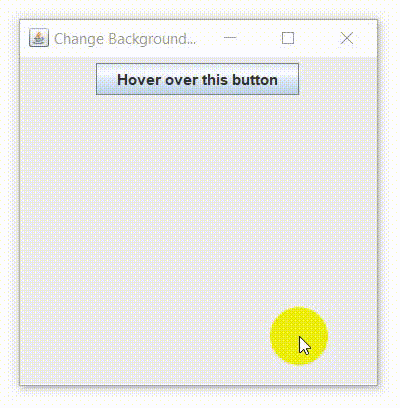