How to add checkbox in menuItem of jMenu in Java Swing
In this tutorial, we are going to see how to add checkbox in menuItem of jMenu in Java Swing. JCheckBoxMenuItem class represents a checkbox that can be included in a menu. CheckBoxMenuItem can be associated with text or a graphical icon. MenuItem can be selected or deselected. MenuItems can be configured and controlled by actions.
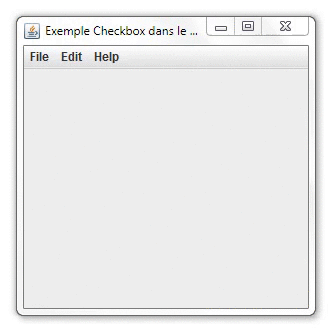
Constructors of JCheckBoxMenuItem class
JCheckBoxMenuItem() | It creates a checkBox in the initially unselected menu with no defined text or icon. |
JCheckBoxMenuItem(Action a) | It creates a checkBox in the menu whose properties are extracted from the provided action. |
JCheckBoxMenuItem(Icon icon) | It creates a checkBox in the initially unselected menu with an icon. |
JCheckBoxMenuItem(String text) | It creates a checkBox in the initially unselected menu with text. |
JCheckBoxMenuItem(String text, boolean b) | It creates a checkBox in the menu with the specified text and selection state. |
JCheckBoxMenuItem(String text, Icon icon) | It creates a checkBox in the initially unselected menu with the specified text and icon. |
JCheckBoxMenuItem(String text, Icon icon, boolean b) | It creates a checkBox in the menu with the specified text, icon, and selection state. |
Commonly used methods:
- getAccessibleContext(): It gets the AccessibleContext associated with this JCheckBoxMenuItem.
- getSelectedObjects(): It returns an array containing the CheckBox label or null if it is not selected.
- getState(): It returns the state of the CheckBox.
- getUIClassID(): It returns the name of the L&F class that renders this component.
- paramString(): It returns a string representation of this JCheckBoxMenuItem.
- setState(boolean b): It sets the state of the CheckBox.
Java Program to add checkbox in menuItem of jMenu:
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class MyJCheckBoxMenuItem { private JFrame f; public MyJCheckBoxMenuItem(){ f = new JFrame("Example of Checkbox in the menu"); f.setSize(300,300); f.setLayout(new GridLayout(3, 1)); f.setVisible(true); } private void showMenu(){ //create a menu bar final JMenuBar bar = new JMenuBar(); //create menus JMenu file = new JMenu("File"); JMenu edit = new JMenu("Edit"); final JMenu help = new JMenu("Help"); //create menu items JMenuItem newF = new JMenuItem("New"); JMenuItem openF = new JMenuItem("Open"); final JCheckBoxMenuItem showhelp = new JCheckBoxMenuItem( "Show Help", true); showhelp.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(showhelp.getState()){ bar.add(help); } else { bar.remove(help); } } }); //add menu items to the main menu file.add(newF); file.add(openF); file.addSeparator(); file.add(showhelp); //add the menu to menu bar bar.add(file); bar.add(edit); bar.add(help); //add the menu bar to the frame f.setJMenuBar(bar); f.setVisible(true); } public static void main(String[] args){ MyJCheckBoxMenuItem obj = new MyJCheckBoxMenuItem(); obj.showMenu(); } }
Output:
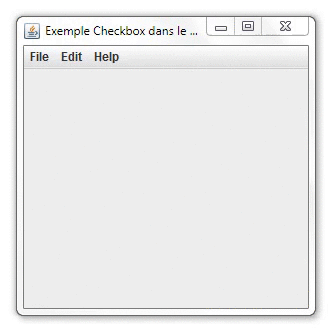