JPasswordField – Java Swing – Example
In this tutorial, we are going to see an example of JPasswordField in Java Swing. JPasswordField is part of javax.swing package. JPasswordField class is a specialized text component for password entry. It allows the edition of a single line of text. JPasswordField inherits from the JTextField class in javax.swing package.
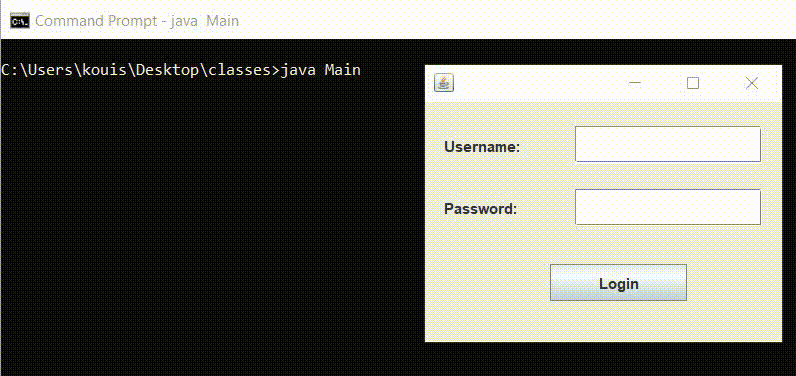
JPasswordField constructors class:
JPasswordField() | Constructor that creates a new Password field. |
JPasswordField(int n) | Constructor that creates a new empty Password field with a specified number of columns. |
JPasswordField(String Password) | Constructor that creates a new empty Password field initialized with the given string. |
JPasswordField(String Password, int n) | Constructor that creates a new empty Password field with the given string and a specified number of columns. |
JPasswordField(Document d, String Password, int n) | Constructor that creates a Password field that uses the given text storage model and the given number of columns. |
Commonly used methods:
- getPassword(): returns the text in JPasswordField.
- getText(): returns the text in JPasswordField.
- getEchoChar(): returns the character used for Echo in JPasswordField.
- setEchoChar(char c): set the Echo character for JPasswordField.
Example of JPasswordField in Java Swing:
import javax.swing.*; import java.awt.event.*; public class Main { public static void main(String[] args) { JFrame frame = new JFrame(); final JPasswordField password = new JPasswordField(); password.setBounds(120, 70, 150, 30); JLabel label1 = new JLabel("Username:"); label1.setBounds(15, 20, 100, 30); JLabel label2 = new JLabel("Password:"); label2.setBounds(15, 70, 100, 30); JButton btn = new JButton("Login"); btn.setBounds(100, 130, 110, 30); final JTextField tf = new JTextField(); tf.setBounds(120, 20, 150, 30); frame.add(password); frame.add(label1); frame.add(label2); frame.add(btn); frame.add(tf); frame.setSize(300,230); frame.setLayout(null); frame.setVisible(true); btn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { System.out.println("Username : "+ tf.getText()); System.out.println("Password: "+ new String(password.getPassword())); } }); } }
Output:
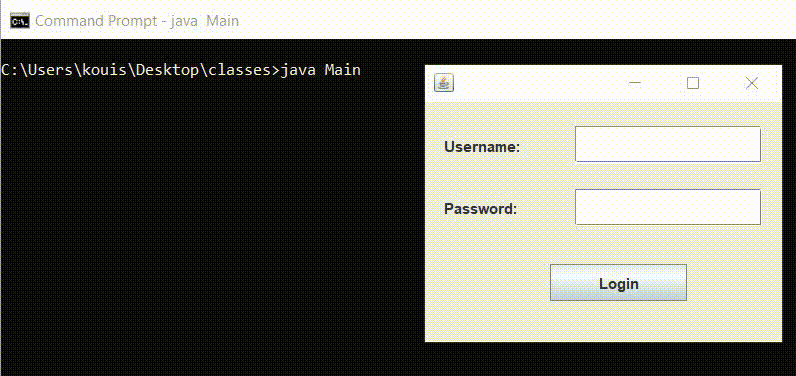