How to Customize JComboBox in Java
In this tutorial, we are going to see how to create a JComboBox component in Java Swing with a custom look, rather than its default appearance.
How to Customize JComboBox in Java
Usually, it is possible to provide a custom GUI implementation for Swing-based components by providing the rendering and editor. For example:
//Create the comboBox JComboBox comboBox = new JComboBox(items); //Define the render comboBox.setRenderer(new MyComboBoxRenderer()); //Define the editor comboBox.setEditor(new MyComboBoxEditor());
Complete Example:
import javax.swing.*; import java.awt.*; public class MyComboBox extends JFrame { public MyComboBox() { //Call the constructor of the parent class JFrame super("Customize JComboBox"); setLayout(new FlowLayout()); //The elements of the comboBoxe String[] items = {"Java", "PHP", "Python", "C++"}; //Create the comboBox JComboBox comboBox = new JComboBox(items); //Define the render comboBox.setRenderer(new MyComboBoxRenderer()); //Define the editor comboBox.setEditor(new MyComboBoxEditor()); //Change the size of the comboBox comboBox.setPreferredSize(new Dimension(130, 25)); //Make the comboBox editable comboBox.setEditable(true); //Add the comboBox to the frame add(comboBox); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(400, 100); //Center the comboBox on the screen setLocationRelativeTo(null); } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new MyComboBox().setVisible(true); } }); } }
The renderer class(MyComboBoxRenderer()) must implement the javax.swing.ListCellRenderer interface. It is used to render the component GUI in its normal state. For example, the following class, MyComboBoxRenderer, implements a simple renderer that is a JLabel:
import javax.swing.*; import java.awt.*; public class MyComboBoxRenderer extends JLabel implements ListCellRenderer { public MyComboBoxRenderer() { setOpaque(true); setFont(new Font("Times New Roman", Font.BOLD | Font.ITALIC, 15)); setBackground(Color.BLACK); setForeground(Color.WHITE); } @Override public Component getListCellRendererComponent(JList l, Object val, int i, boolean isSelected, boolean cellHasFocus) { setText(val.toString()); return this; } }
As we can see, the renderer class must override the getListCellRendererComponent() method that is defined by the ListCellRenderer interface. The method must return a subclass of the Component class that is rendered as a list item in the ComboBox. In the example above, it returns an instance of JLabel class, and the results in a drop-down list box look like this:
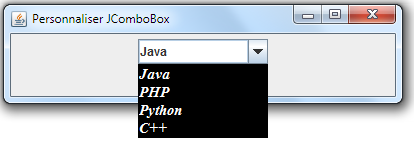