JButton – Java Swing – Example
In this tutorial, we are going to see an example of JButton in Java Swing. JButton is a component of Java Swing. JButton class is used to create a labeled button with a platform-independent implementation. The application causes an action when the button is clicked. It can be configured to have different actions, using the Event Listener. JButton inherits from the AbstractButton class.
JButton constructors class:
JButton() | It creates a button without text or icon. |
JButton(String s) | It creates a button with the specified text. |
JButton(Icon i) | It creates a button with the specified icon object. |
Commonly used methods of JButton class:
- void setText(String s) : It is used to set the text specified on the button.
- String getText() : It is used to return the text of the button.
- void setEnabled(boolean b) : It is used to enable or disable the button.
- void setIcon(Icon b) : It is used to set the icon on JButton.
- Icon getIcon() : It is used to get the button icon.
- void setMnemonic(int a) : It is used to set the mnemonic on the button.
- void addActionListener(ActionListener a) : It is used to add action listener to this object.
Example 1 of JButton in Java Swing:
import javax.swing.*; public class Main { public static void main(String[] args) { //Create a new frame JFrame frame = new JFrame("JButton Example"); //Create button JButton btn = new JButton("Click here"); //Set button position btn.setBounds(100,100,100,40); //Add button to frame frame.add(btn); frame.setSize(300,300); frame.setLayout(null); frame.setVisible(true); } }
Output:
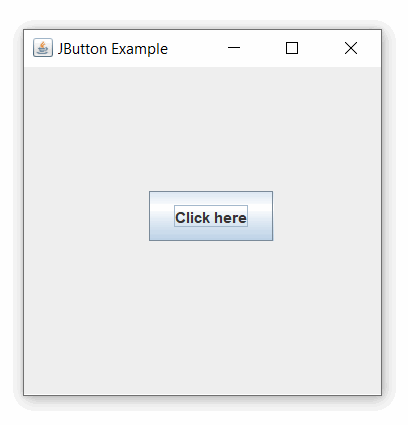
Example 2 of JButton in Java Swing with ActionListener:
import javax.swing.*; import java.awt.event.*; public class Main { public static void main(String[] args) { //Create a new frame JFrame frame = new JFrame("JButton Example"); final JTextArea textArea = new JTextArea(); textArea.setBounds(50,50, 180,20); //Create button JButton btn = new JButton("Click here"); //Set button position btn.setBounds(90,100,100,40); btn.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ textArea.setText("Welcome to StackHowTo!"); } }); //Add JButton and JtextArea to the frame frame.add(btn); frame.add(textArea); frame.setSize(300,300); frame.setLayout(null); frame.setVisible(true); } }
Output:
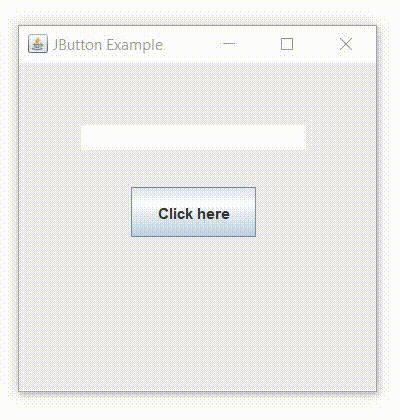