JList – Java Swing – Example
In this tutorial, we are going to see an example of JList in Java Swing. JList is part of the Java Swing package. JList is a component that displays a set of objects and allows the user to select one or more items. JList inherits from the JComponent class. JList is an easy way to display an array of vectors.
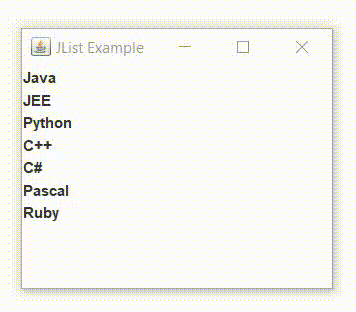
JList constructors class:
JList() | Create an empty list |
JList(E[] l) | Create a new list with the elements of the array. |
JList(ListModel d) | Create a new list with the specified list model |
JList(Vector l) | Create a new list with the elements of the vector |
Example of JList in Java Swing:
import javax.swing.*; public class JListExample extends JFrame { private JList<String> langages; public JListExample() { //create the model and add elements DefaultListModel<String> model = new DefaultListModel<>(); model.addElement("Java"); model.addElement("JEE"); model.addElement("Python"); model.addElement("C++"); model.addElement("C#"); model.addElement("Pascal"); model.addElement("Ruby"); //create the list of languages langages = new JList<>(model); add(langages); this.setTitle("JList Example"); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setSize(200,200); this.setLocationRelativeTo(null); this.setVisible(true); } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new JListExample(); } }); } }
Output:
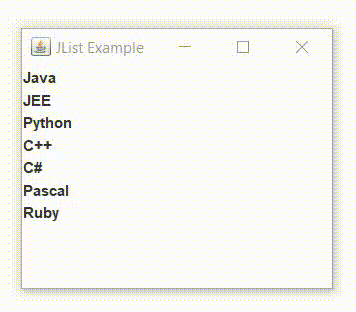