JComponent – Java Swing – Example
In this tutorial, we are going to see an example of JComponent in Java Swing. JComponent class is the base class for all Swing components, except for top-level containers. Swing components whose names start with “J” are descendants of JComponent class. For example, JButton, JScrollPane, JPanel, JTable, etc. But, JFrame and JDialog do not inherit from JComponent class because they belong to higher-level containers.
JComponent class inherits from Container class which itself inherits from Component. The Container class supports adding components to the container.
Example of JComponent in Java Swing
import java.awt.*; import javax.swing.*; class ComponentTest extends JComponent { public void paint(Graphics g) { g.setColor(Color.red); g.fillRect(40, 40, 100, 100); } } public class Main { public static void main(String[] arguments) { ComponentTest c = new ComponentTest(); // create a basic JFrame JFrame.setDefaultLookAndFeelDecorated(true); JFrame frame = new JFrame("JComponent Example"); frame.setSize(300,300); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // add JComponent to the frame frame.add(c); frame.setVisible(true); } }
Output:
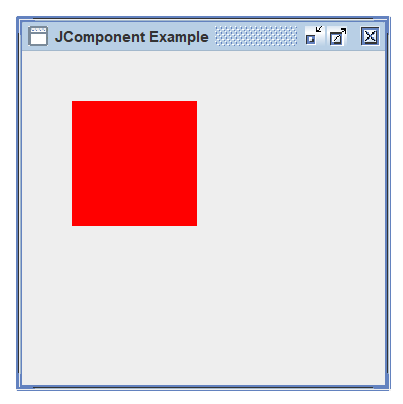