How to Delete a Selected Row from JTable in Java
In this tutorial, we are going to see how to delete a selected row from a JTable in Java. JTable is a subclass of JComponent class for displaying complex data structures. JTable can follow the Model View Controller (MVC) design pattern to display data in rows and columns. JTable can generate ListSelectionListener, TableColumnModelListener, TableModelListener, CellEditorListener and RowSorterListener interfaces. We can remove a selected row from a JTable by using removeRow() method of DefaultTableModel class.
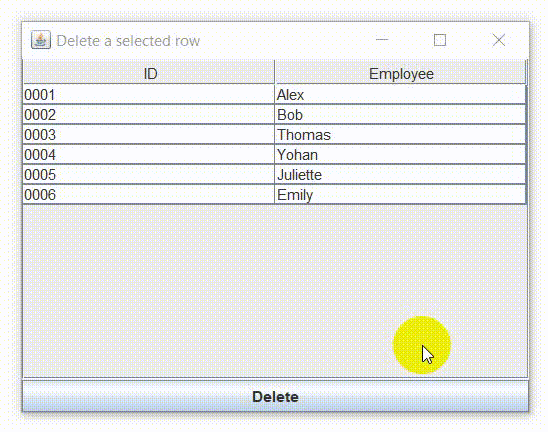
How to Delete a Selected Row from JTable in Java
import java.awt.*; import java.awt.event.*; import javax.swing.*; import javax.swing.table.*; public class DeleteRow extends JFrame { private JTable table; private JButton btn; private DefaultTableModel tablemodel; private String[] columns; private Object[][] rows; public DeleteRow() { setTitle("Delete a selected row"); columns = new String[] {"ID", "Employee"}; rows = new Object[][] { {"0001", "Alex"}, {"0002", "Bob"}, {"0003", "Thomas"}, {"0004", "Yohan"}, {"0005", "Juliette"}, {"0006", "Emily"} }; tablemodel = new DefaultTableModel(rows, columns); table = new JTable(tablemodel); table.setSelectionMode(ListSelectionModel.SINGLE_SELECTION); btn = new JButton("Delete"); btn.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent ae) { // check the selected row first if(table.getSelectedRow() != -1) { // remove the selected row from the table model tablemodel.removeRow(table.getSelectedRow()); JOptionPane.showMessageDialog(null, "Deleted successfully"); } } }); add(new JScrollPane(table), BorderLayout.CENTER); add(btn, BorderLayout.SOUTH); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(420, 320); setLocationRelativeTo(null); setVisible(true); } public static void main(String args[]) { new DeleteRow(); } }
Output:
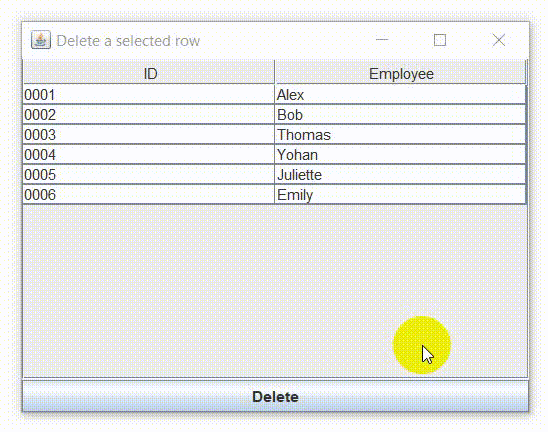