How to Increase Row Height in JTable
In this tutorial, we are going to see how to increase row height in JTable. When working with JTable in Swing, we sometimes need the table to have rows of different heights, other than the default values provided by JTable component. This allows us to enlarge the cells of JTable.
Let’s look at an example of a Swing program, how to change the default value of the height of a row in a JTable:
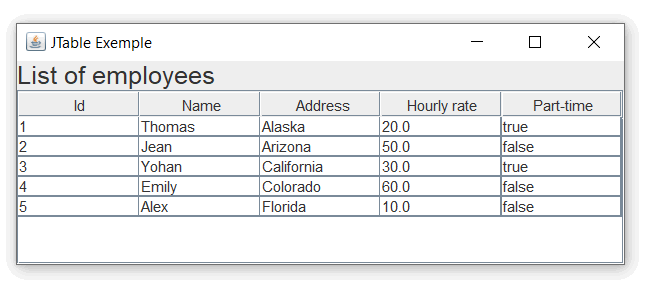
How to Increase Row Height in JTable
This can be done easily by using these two methods of JTable class:
- setRowHeight(int row, int height): defines the height (in pixels) of a single line.
- setRowHeight(int height): defines the height (in pixels) of all the lines of the JTable defined before.
For example, the following code sets the height of all lines to 30 pixels:
table.setRowHeight(30);
Output:
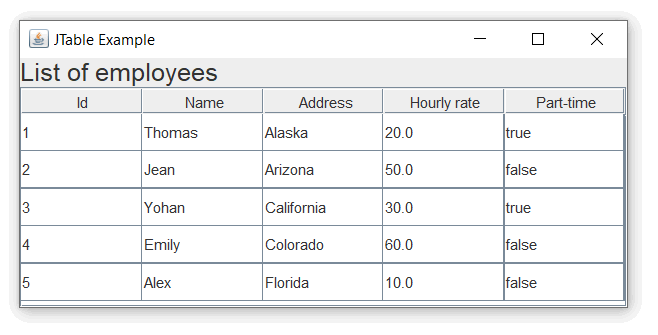
We can define the height of a single line, for example, the third line (index = 2) will have a height of 50 pixels:
table.setRowHeight(2, 50);
Output:
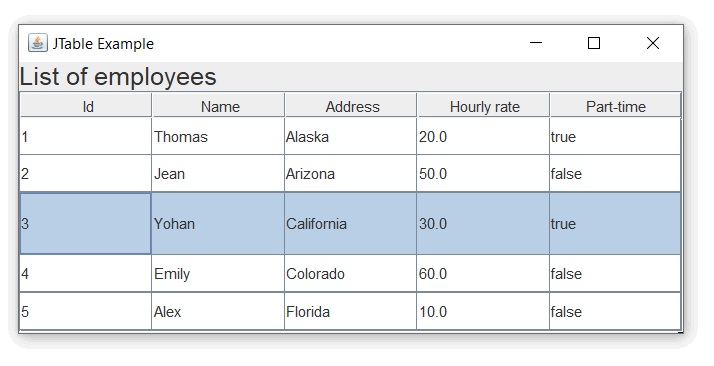
Complete Example:
import javax.swing.*; import java.awt.*; public class Main { public static void main(String[] args) { //create a frame final JFrame frame = new JFrame("JTable Example"); //Headers for JTable String[] columns = new String[] { "Id", "Name", "Address", "Hourly rate", "Part-time" }; //data for JTable in a 2D table Object[][] data = new Object[][] { {1, "Thomas", "Alaska", 20.0, true }, {2, "Jean", "Arizona", 50.0, false }, {3, "Yohan", "California", 30.0, true }, {4, "Emily", "Colorado", 60.0, false }, {5, "Alex", "Florida", 10.0, false }, }; //create a JTable with data JTable table = new JTable(data, columns); table.setRowHeight(30); table.setRowHeight(2, 50); JScrollPane scroll = new JScrollPane(table); table.setFillsViewportHeight(true); JLabel labelHead = new JLabel("List of employees"); labelHead.setFont(new Font("Arial",Font.TRUETYPE_FONT,20)); frame.getContentPane().add(labelHead,BorderLayout.PAGE_START); //add table to frame frame.getContentPane().add(scroll,BorderLayout.CENTER); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(500, 180); frame.setVisible(true); } }
Output:
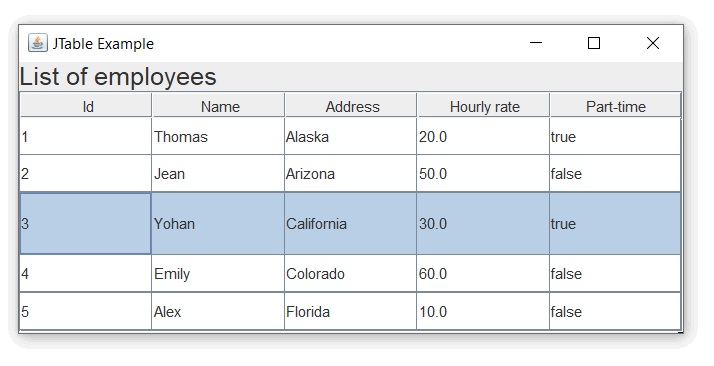