JFileChooser – Java Swing – Example
In this tutorial, we are going to see an example of JFileChooser in Java Swing. JFileChooser is part of the java Swing package. JFileChooser is a simple and efficient way to invite the user to choose a file or a directory. In this tutorial we will discover how to use JFileChooser in Java Swing.
JFileChooser constructors class:
JFileChooser() | Empty constructor that points to the user’s default directory. |
JFileChooser(String) | Uses the given path |
JFileChooser(File) | Use the given file as path |
JFileChooser(FileSystemView) | Uses the given FileSystemView |
JFileChooser(String, FileSystemView) | Uses the given path and FileSystemView |
JFileChooser(File, FileSystemView) | Uses the given current directory and FileSystemView |
Example 1: Open or save a file
import java.io.File; import javax.swing.JFileChooser; import javax.swing.filechooser.FileSystemView; public class Main { public static void main(String[] args) { JFileChooser choose = new JFileChooser( FileSystemView .getFileSystemView() .getHomeDirectory() ); // Open the file int res = choose.showOpenDialog(null); // Save the file // int res = choose.showSaveDialog(null); if (res == JFileChooser.APPROVE_OPTION) { File file = choose.getSelectedFile(); System.out.println(file.getAbsolutePath()); } } }
Note that the two methods showOpenDialog() and showSaveDialog() are similar, what makes the difference is how the developer handles each of them.
Output:
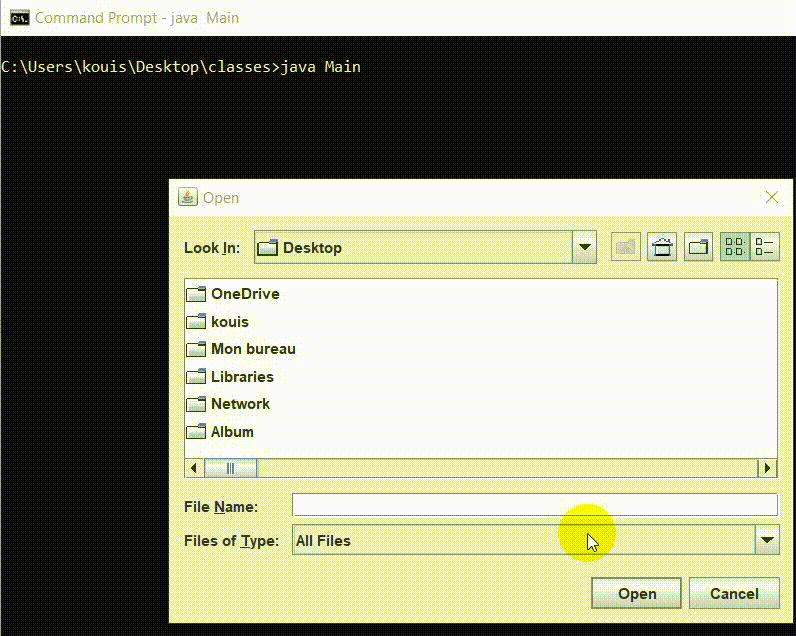
Example 2: Select files or directories
With this method, we can limit the user to select either directories only (JFileChooser.DIRECTORIES_ONLY) or files only (JFileChooser.FILES_ONLY) or files and directories (JFileChooser.FILES_AND_DIRECTORIES). The default value is FILES_ONLY. Here is an example that implements JFileChooser.DIRECTORIES_ONLY:
import javax.swing.JFileChooser; import javax.swing.filechooser.FileSystemView; public class Main { public static void main(String[] args) { JFileChooser choose = new JFileChooser( FileSystemView .getFileSystemView() .getHomeDirectory() ); choose.setDialogTitle("Choose a directory: "); choose.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY); int res = choose.showSaveDialog(null); if(res == JFileChooser.APPROVE_OPTION) { if(choose.getSelectedFile().isDirectory()) { System.out.println("You have selected the directory: "+ choose.getSelectedFile()); } } } }
Output:
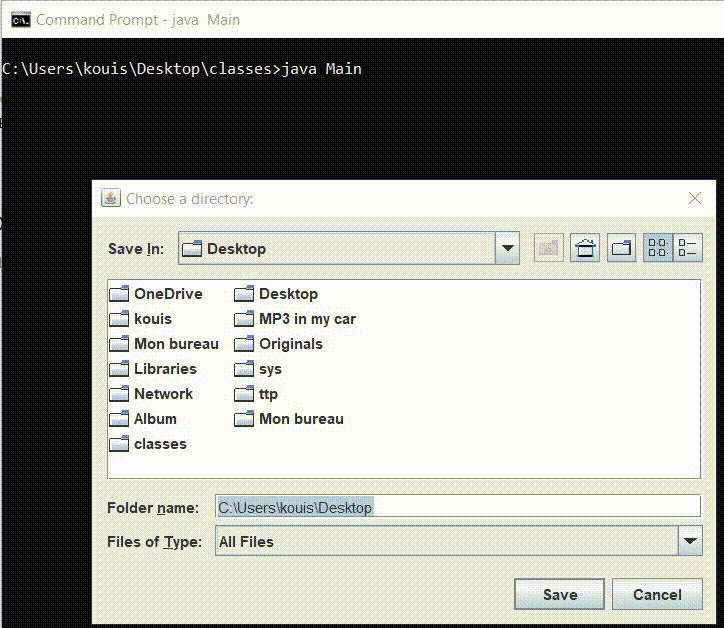
Example 3: Filter files
If your program requires jpg and gif images, it would be more practical to limit the user’s selection to that. The example below shows how to achieve this using the custom FileNameExtensionFilter class:
import javax.swing.JFileChooser; import javax.swing.filechooser.*; public class Main { public static void main(String[] args) { JFileChooser choose = new JFileChooser( FileSystemView .getFileSystemView() .getHomeDirectory() ); choose.setDialogTitle("Select an image"); choose.setAcceptAllFileFilterUsed(false); FileNameExtensionFilter filter = new FileNameExtensionFilter("JPG and GIF images", "jpg", "gif"); choose.addChoosableFileFilter(filter); int res = choose.showOpenDialog(null); if (res == JFileChooser.APPROVE_OPTION) { System.out.println(choose.getSelectedFile().getPath()); } } }
Output:
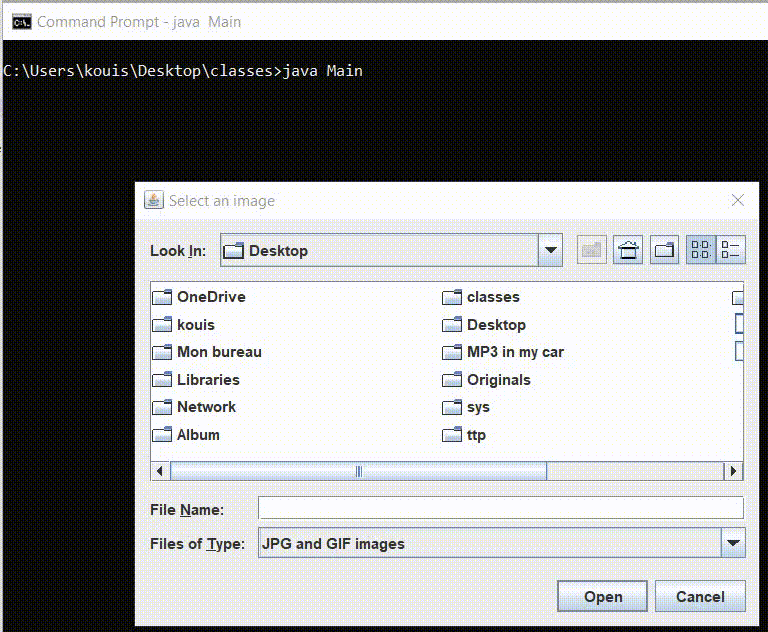
As you can see, the user is not allowed to choose anything else. The directory shown above also contains other types of images, but only jpg and gif are displayed to the user.