How to Hide a Column in JTable
In this tutorial, we are going to see how to hide a column in JTable. JTable is a flexible Swing component that is very well suited to display data in a tabular format. In this tutorial, you will learn some techniques to hide a column in a JTable or to make a column in a JTable invisible.
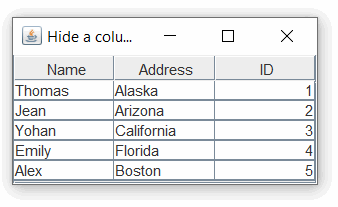
[st_adsense]
In the example below, we have three columns “Name, address, ID”, and we have hidden the last column “ID”. In the console we print the hidden data using TableModel.
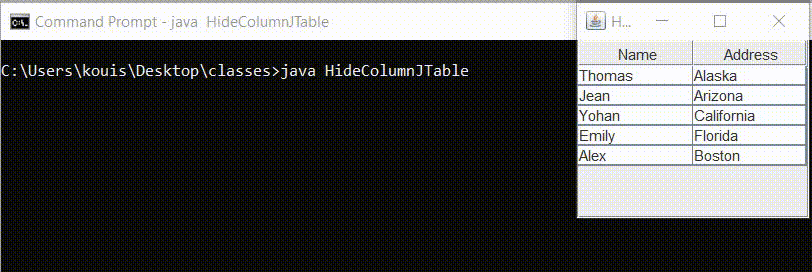
Method 1 :
You can use removeColumn() method.
Syntax:
removeColumn(TableColumn tcol)
This method is used to delete a column from JTable. It takes a TableColumn object that represents all attributes of a column in a JTable. tcol: It indicates the TableColumn that will be deleted.
Example:
//remove the second column table.removeColumn(table.getColumnModel().getColumn(1));
To get data from the model:
table.getModel().getValueAt(table.getSelectedRow(),1);
One thing to note is, when retrieving data, it must be extracted from the model and not from the table.
[st_adsense]
Method 2 :
You can set the column width to 0, so that it is invisible, for this we will use the following methods.
- setMinWidth()
- setMaxWidth()
- setWidth()
Example:
table.getColumnModel().getColumn(4).setMinWidth(0); table.getColumnModel().getColumn(4).setMaxWidth(0); table.getColumnModel().getColumn(4).setWidth(0);
Method 3 :
To hide a column (or more) in a JTable, do not give the column name. To get the hidden data, you must use the TableModel.
[st_adsense]
Example:
import javax.swing.*; import java.awt.*; import javax.swing.table.*; import javax.swing.event.*; import java.awt.event.*; public class HideColumnJTable implements ListSelectionListener { JTable table; public HideColumnJTable() { JFrame f = new JFrame("Hide a column from JTable"); // We only specify 2 columns, the last "ID" is hidden String[] columns = new String[] { "Name", "Address", }; Object[][] data = new Object[][] { {"Thomas", "Alaska", 1 }, {"Jean", "Arizona", 2 }, {"Yohan", "California", 3 }, {"Emily", "Florida", 4 }, {"Alex", "Boston", 5 }, }; TableModel model = new AbstractTableModel() { public int getColumnCount() { return columns.length; } public int getRowCount() { return data.length; } public Object getValueAt(int row, int col) { return data[row][col]; } public String getColumnName(int column) { return columns[column]; } public Class getColumnClass(int col) { return getValueAt(0,col).getClass(); } public void setValueAt(Object aValue, int row, int column) { data[row][column] = aValue; } }; table = new JTable(model); ListSelectionModel listModel = table.getSelectionModel(); listModel.setSelectionMode(ListSelectionModel.SINGLE_SELECTION); listModel.addListSelectionListener(this); JScrollPane scroll = new JScrollPane(table); scroll.setPreferredSize(new Dimension(300, 300)); f.getContentPane().add(scroll); f.setSize(200, 180); f.setVisible(true); } public void valueChanged(ListSelectionEvent e) { int[] sel; Object value; if (!e.getValueIsAdjusting()) { sel = table.getSelectedRows(); if (sel.length > 0) { for (int i=0; i < 3; i++) { // get data from the table TableModel tm = table.getModel(); value = tm.getValueAt(sel[0],i); System.out.print(value + " "); } System.out.println(); } } } public static void main(String[] args) { new HideColumnJTable(); } }
Output:
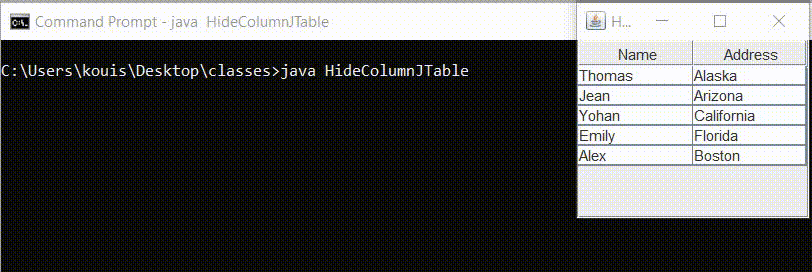
[st_adsense]