JTree – Java Swing – Example
In this tutorial, we are going to see an example of JTree in Java Swing. JTree class is used to display tree-structured or hierarchical data. JTree is a complex component. It has a “root node” at the top that is the parent of all nodes in the tree. It inherits from the JComponent class.
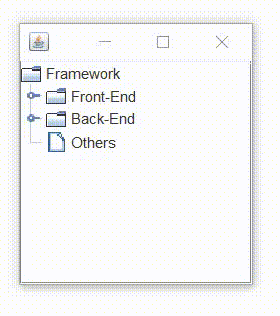
JTree constructors class:
JTree() | Create a JTree. |
JTree(Object[] value) | Creates a JTree with each element of the specified array as a child of a new root node. |
JTree(TreeNode root) | Creates a JTree with the specified TreeNode as root, which displays the root node. |
Example of JTree in Java Swing:
Let’s look at how to build a simple JTree. Let’s say we want to display the list of backend and frontend frameworks in a hierarchical way.
The node is represented by the TreeNode class which is an interface. The MutableTreeNode interface inherits this interface which represents a mutable node. The Swing API provides an implementation of this interface in the form of DefaultMutableTreeNode class.
We will use DefaultMutableTreeNode class to represent our node. This class is provided in the Swing API and we can use it to represent our nodes. This class has a handy add() method that takes an instance of MutableTreeNode.
So first we’ll create the root node. And then we can recursively add nodes to that root.
import javax.swing.*; import javax.swing.tree.DefaultMutableTreeNode; public class MyTree { MyTree() { JFrame f = new JFrame(); //root node DefaultMutableTreeNode framework = new DefaultMutableTreeNode("Framework"); //inner node 1 DefaultMutableTreeNode front = new DefaultMutableTreeNode("Front-End"); //inner node 2 DefaultMutableTreeNode back = new DefaultMutableTreeNode("Back-End"); //leaf DefaultMutableTreeNode others = new DefaultMutableTreeNode("Others"); //Add inner node 1 to the root node framework.add(front); //Add leaves to node 1 DefaultMutableTreeNode angular = new DefaultMutableTreeNode("AngularJS"); DefaultMutableTreeNode react = new DefaultMutableTreeNode("React.js"); DefaultMutableTreeNode meteor = new DefaultMutableTreeNode("Meteor.js"); DefaultMutableTreeNode ember = new DefaultMutableTreeNode("Ember.js "); front.add(angular); front.add(react); front.add(meteor); front.add(ember); //Add inner node 2 to the root node framework.add(back); //Add leaves to node 2 DefaultMutableTreeNode nodejs = new DefaultMutableTreeNode("NodeJS"); DefaultMutableTreeNode express = new DefaultMutableTreeNode("Express"); back.add(nodejs); back.add(express); //Add the last leaf to the root node framework.add(others); JTree jt = new JTree(framework); f.add( new JScrollPane(jt) //Add a scroll bar ); f.setSize(200,200); f.setVisible(true); } public static void main(String[] args) { new MyTree(); } }
Output:
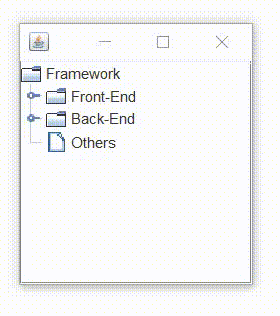