SpringLayout – Java Swing – Example
In this tutorial, we are going to see an example of SpringLayout in Java Swing. SpringLayout organizes the elements of its associated container according to a set of constraints. Constraints are nothing more than horizontal and vertical distance between two components. All constraints are represented by a SpringLayout.Constraint object.
Each element of a SpringLayout container, as well as the container itself, has exactly one set of constraints associated with it.
Each edge position depends on the position of the other edge. If a constraint is added to create a new edge, the previous binding is removed. SpringLayout does not automatically define the location of the components it manages.
SpringLayout class constructor:
- SpringLayout(): used to build a new SpringLayout class.
Commonly used methods of SpringLayout:
- addLayoutComponent(Component c, Object obj): If the constraints are an instance of SpringLayout.Constraints, associate the constraints to the specified component.
- getLayoutAlignmentX(Container c): used to return 0.5f (centered).
- getLayoutAlignmentY(Container c): used to return 0.5f (centered).
- getConstraint((String str, Component c): returns the spring controlling the distance between the specified edge of the component and the top or left edge of its parent.
- getConstraint(Component c): returns the constraints of the specified component.
- layoutContainer(Container parent): used to arrange the specified container.
Example of SpringLayout in Java Swing:
import java.awt.*; import javax.swing.*; public class Main { public static void main(String args[]) { JFrame f = new JFrame("SpringLayout Example"); Container content = f.getContentPane(); SpringLayout layout = new SpringLayout(); content.setLayout(layout); Component lab = new JLabel("Label :"); Component text = new JTextField(15); content.add(lab); content.add(text); layout.putConstraint(SpringLayout.WEST, lab, 20, SpringLayout.WEST, content); layout.putConstraint(SpringLayout.NORTH, lab, 20, SpringLayout.NORTH, content); layout.putConstraint(SpringLayout.NORTH, text, 20, SpringLayout.NORTH, content); layout.putConstraint(SpringLayout.WEST, text, 20, SpringLayout.EAST, lab); f.setSize(300, 100); f.setVisible(true); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } }
Output:
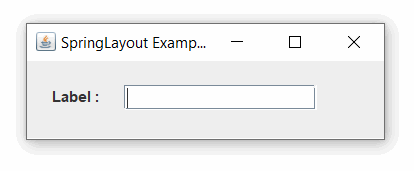