JSpinner – Java Swing – Example
In this tutorial, we are going to see an example of JSpinner in Java Swing. JSpinner is part of javax.swing package. JSpinner contains a single line of input that can be a number or an object in an ordered sequence. The user can manually enter data into the spinner’s text field. The spinner is sometimes preferred because it does not need a drop-down list. Spinners contain up/down arrows to display the previous and next item when pressed.
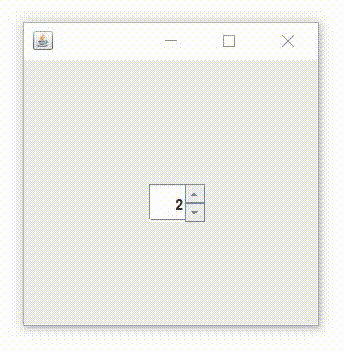
JSpinner constructors class:
JSpinner() | Creates an empty spinner with an initial value set to zero and no constraints. |
JSpinner(SpinnerModel model) | Creates a spinner with a specified spinner template transmitted as argument. |
Commonly used methods:
- setValue(Object v): Defines the value of the spinner on the object transmitted in argument.
- getValue(): Returns the current value of the spinner.
- getPreviousValue(): Returns the previous value of the spinner.
- getNextValue(): Returns the next value of the spinner.
- SpinnerListModel(List l): Creates a spinner model with elements from the list l. This spinner model can be used to define as a spinner model.
- SpinnerNumberModel(int val, int max, int min, int step): Returns a spinner pattern whose initial value is set to ‘val’, with a minimum and maximum value, and a step value defined.
Example of JSpinner in Java Swing:
import javax.swing.*; import javax.swing.event.*; public class Main { public static void main(String[] args) { JFrame frame = new JFrame(); final JLabel label = new JLabel(); label.setHorizontalAlignment(JLabel.CENTER); label.setSize(250,100); SpinnerModel model = new SpinnerNumberModel( 2, //initial value 0, //minimum value 20, //maximum value 1 //step ); JSpinner sp = new JSpinner(model); sp.setBounds(100,100,45,30); frame.add(sp); frame.add(label); frame.setSize(250,250); frame.setLayout(null); frame.setVisible(true); //when the up/down arrows is pressed sp.addChangeListener(new ChangeListener() { public void stateChanged(ChangeEvent e) { label.setText("Value : " + ((JSpinner)e.getSource()).getValue()); } }); } }
Output:
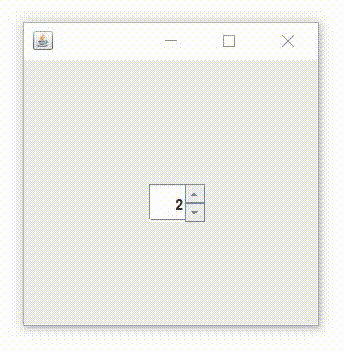