Dialog boxes – JOptionPane – Java Swing – Example
In this tutorial, we are going to see an example of JOptionPane in Java Swing. JOptionPane class is used to provide standard dialog boxes such as the message box, confirmation box, and input box. These dialog boxes are used to display information or get information from the user. JOptionPane class inherits from JComponent class.
JOptionPane constructors class:
JOptionPane() | It is used to create an instance of JOptionPane with a test message. |
JOptionPane(Object message) | It is used to create an instance of JOptionPane to display a message. |
JOptionPane(Object message, int messageType) | It is used to create an instance of JOptionPane to display a message with the specified message type and default options. |
Commonly used methods:
- createDialog(String title) : It is used to create and return a new JDialog without parent with the specified title.
- showMessageDialog(Component parentComponent, Object message) : It is used to create an information message box called “Message”..
- showMessageDialog(Component parentComponent, Object message, String title, int messageType) : It is used to create a message box with a given title and message type.
- showConfirmDialog(Component parentComponent, Object message) : It is used to create a dialog box with Yes, No and Cancel options.
- showInputDialog(Component parentComponent, Object message) : It is used to display a question-message box requesting user input.
- void setInputValue(Object newValue) : It is used to set the value entered by the user.
Example 1: showMessageDialog()
import javax.swing.*; public class MyJOptionPane { MyJOptionPane() { JFrame frame = new JFrame(); JOptionPane.showMessageDialog(frame,"Welcome to StackHowTo!"); } public static void main(String[] args) { new MyJOptionPane(); } }
Output:
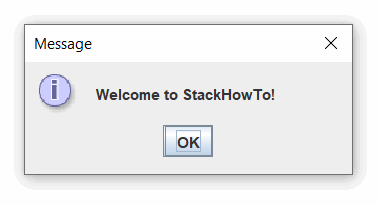
Example 2: showMessageDialog()
import javax.swing.*; public class MyJOptionPane { MyJOptionPane() { JFrame frame = new JFrame(); JOptionPane.showMessageDialog( frame, "Connection Failure!", "Alert", JOptionPane.WARNING_MESSAGE ); } public static void main(String[] args) { new MyJOptionPane(); } }
Output:
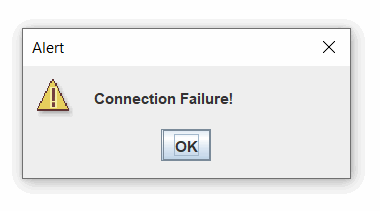
Example 3: showInputDialog()
import javax.swing.*; public class MyJOptionPane { MyJOptionPane() { JFrame frame = new JFrame(); String nom = JOptionPane.showInputDialog(frame,"Enter your name:"); } public static void main(String[] args) { new MyJOptionPane(); } }
Output:
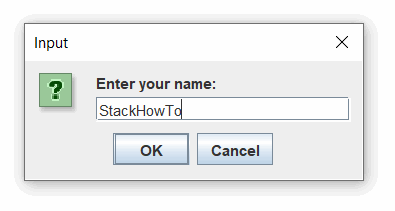
Example 4: showConfirmDialog()
import javax.swing.*; import java.awt.event.*; public class MyJOptionPane extends WindowAdapter { JFrame frame; MyJOptionPane() { frame = new JFrame(); frame.addWindowListener(this); frame.setSize(250, 250); frame.setLayout(null); frame.setVisible(true); frame.setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE); } public void windowClosing(WindowEvent e) { int res = JOptionPane.showConfirmDialog(frame,"Are you sure?"); if(res == JOptionPane.YES_OPTION) { frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } public static void main(String[] args) { new MyJOptionPane(); } }
Output:
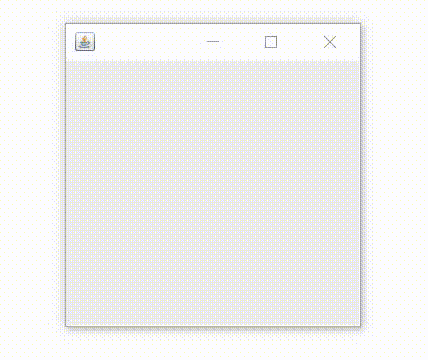