ActionListener – Java Swing – Example
In this tutorial, we are going to see an example of ActionListener in Java Swing. Java ActionListener is notified every time you click the button. It is notified by ActionEvent. The ActionListener interface exists in java.awt.event package. It has only one method actionPerformed(). This method is invoked automatically every time you click the button.
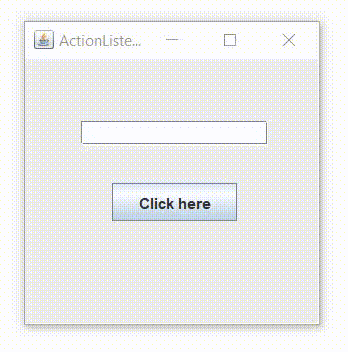
How to implement ActionListener
First you should implement ActionListener interface. If you implement ActionListener interface, you need to follow 3 steps:
1) Implement ActionListener interface:
public class MyJButtonActionListener implements ActionListener
2) Register the component with the Listener:
component.addActionListener(instanceOfMyJButtonActionListener);
3) Override the method actionPerformed():
public void actionPerformed(ActionEvent e){ // put the code here... }
Example : ActionListener in Java Swing
import javax.swing.*; import javax.swing.event.*; import java.awt.event.*; //1st step public class MyJButtonActionListener implements ActionListener { private static JTextField text; public static void main(String[] args) { JFrame frame = new JFrame("ActionListener Example"); text = new JTextField(); text.setBounds(45,50,150,20); JButton btn = new JButton("Click here"); btn.setBounds(70,100,100,30); MyJButtonActionListener instance = new MyJButtonActionListener(); //2nd step btn.addActionListener(instance); frame.add(btn); frame.add(text); frame.setSize(250,250); frame.setLayout(null); frame.setVisible(true); } //3rd step public void actionPerformed(ActionEvent e){ text.setText("Welcome to StackHowTo"); } }
Output:
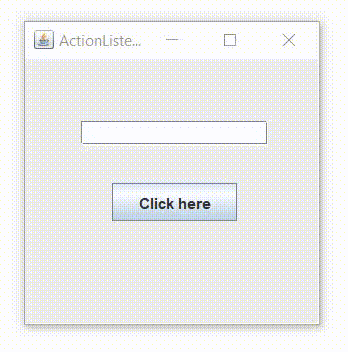