How to add and remove items in JComboBox in Java
In this tutorial, we are going to see how to add and remove items in JComboBox in Java. JComboBox is part of the Java Swing package. JComboBox inherits from the JComponent class. JComboBox displays a contextual menu which shows a list and the user can select an option in this specified list.
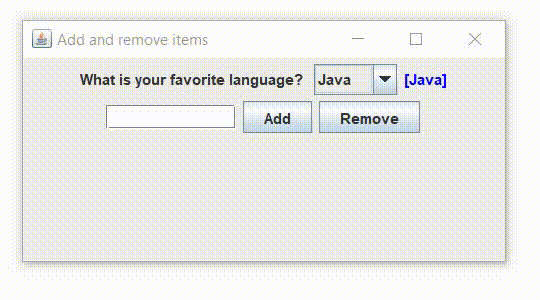
Java Program to Add and Remove Items in JComboBox:
import javax.swing.*; import java.awt.*; import java.awt.event.*; class ComboBoxExample extends JFrame implements ItemListener, ActionListener { // frame static JFrame frame; // combobox static JComboBox combobox; // label static JLabel l1, l2; // textfield static JTextField text; public static void main(String[] args) { // create a new frame frame = new JFrame("Add and remove items"); // create an object ComboBoxExample obj = new ComboBoxExample(); // array of strings containing languages String langs[] = { "Java", "PHP", "Python", "C++", "Ruby" }; // create a checkbox combobox = new JComboBox(langs); // create textfield text = new JTextField(10); // create add and remove buttons JButton b1 = new JButton("Add"); JButton b2 = new JButton("Remove"); // add action listener b1.addActionListener(obj); b2.addActionListener(obj); // add ItemListener combobox.addItemListener(obj); // create labels l1 = new JLabel("What is your favorite language? "); l2 = new JLabel("[Java]"); // set text color l2.setForeground(Color.blue); // create a new panel JPanel p = new JPanel(); // add combobox, textfield, button and labels to the panel p.add(l1); p.add(combobox); p.add(l2); p.add(text); p.add(b1); p.add(b2); // add panel to frame frame.add(p); // set the frame size frame.setSize(400, 200); frame.setLayout(new GridLayout(2, 1)); frame.show(); } // if the button is pressed public void actionPerformed(ActionEvent e) { String str = e.getActionCommand(); if (str.equals("Add")) { combobox.addItem(text.getText()); } else { combobox.removeItem(text.getText()); } } public void itemStateChanged(ItemEvent e) { // check if the state of the combobox is modified if (e.getSource() == combobox) { l2.setText(" ["+combobox.getSelectedItem()+"]"); } } }
Output:
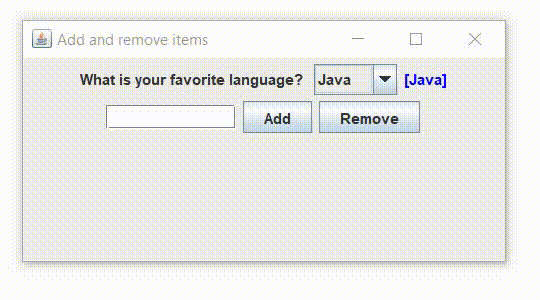