How to Change Font Size and Font Style of a JLabel
In this tutorial, we are going to see how to change the font size and font style of a JLabel in Java Swing. JLabel is an area to display short text or an image, or both, it is a basic GUI component defined in the Java Swing library. A label does not react to input events. Therefore, it cannot get keyboard focus.
How to change font size of the JLabel
JLabel label = new JLabel("This is a label!"); label.setFont(new Font("Serif", Font.BOLD, 20)); JFrame frame = new JFrame(); frame.add(label); frame.setVisible(true);
Output:
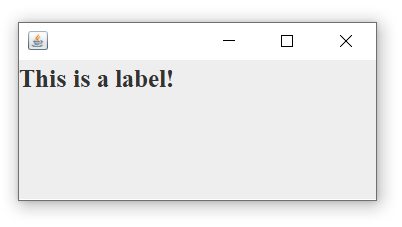
How to change the color of the JLabel
label.setForeground(Color.RED);
Output:
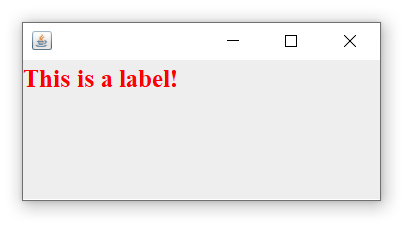
How to change the background color of the JLabel
label.setBackground(Color.ORANGE); label.setOpaque(true);
Output:
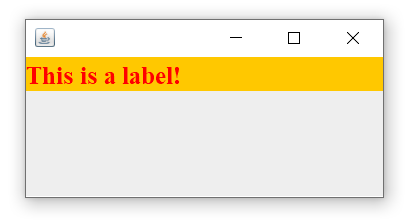
How to center the text in a JLabel
label = new JLabel("This is a label!", SwingConstants.CENTER);
Output:
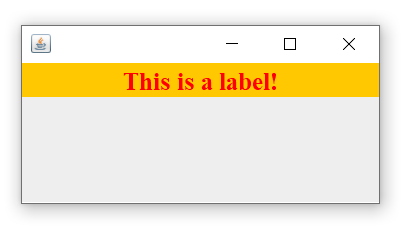
Complete Example: How to Change Font Size and Font Style of a JLabel
import java.awt.*; import javax.swing.*; public class StyleJLabel { StyleJLabel() { JFrame frame = new JFrame(); frame.setLayout(new GridLayout(4,1)); JLabel label = new JLabel("This is a label!", SwingConstants.CENTER); label.setFont(new Font("Serif", Font.BOLD, 20)); label.setForeground(Color.RED); label.setBackground(Color.ORANGE); label.setOpaque(true); frame.add(label); frame.setSize(300,150); frame.setVisible(true); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { new StyleJLabel(); } }
Output:
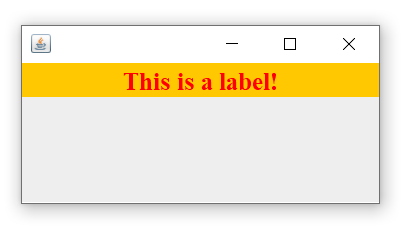