How to Disable JTextArea and JtextField
In this tutorial, we are going to see how to disable JTextArea and JtextField. There are two ways to do this, either by disabling the component or by preventing modification.
To disable JtextField/JTextArea, call the method setEnabled() and pass the value “false” as parameter.
JTextField textField = new JTextField(); textField.setEnabled(false); JTextArea textArea = new JTextArea(5, 50); textArea.setEnabled(false);
To make JtextField/JTextArea uneditable, call the method setEnabled() and pass the value “false” as parameter.
JTextField textField = new JTextField(); textField.setEditable(false); JTextArea textArea = new JTextArea(5, 50); textArea.setEditable(false);
Complete Example: How to Disable JTextArea and JtextField
import java.awt.*; import javax.swing.*; public class DisableJTextField { DisableJTextField() { JFrame frame = new JFrame(); frame.setLayout(new GridLayout(4,1)); JTextField textField = new JTextField("JTextField : Welcome to StackHowTo!"); textField.setEnabled(false); //text.setEditable(false); JTextArea textArea = new JTextArea(5, 50); textArea.setText("JTextArea : Welcome to StackHowTo!"); textArea.setEnabled(false); //textArea.setEditable(false); frame.add(textField); frame.add(textArea); frame.setSize(300,300); frame.setVisible(true); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { new DisableJTextField(); } }
Output:
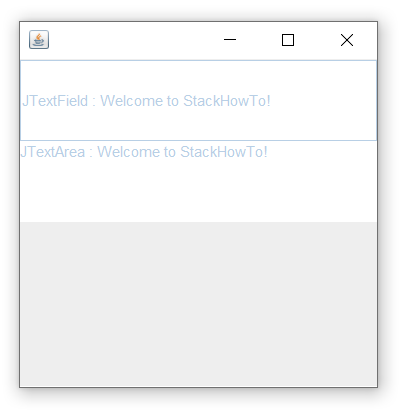