How to get the selected item of a JComboBox in Java
In this tutorial, we are going to see how to get the selected item of a JComboBox in Java. JComboBox is part of Java Swing package. JComboBox inherits from JComponent class. JComboBox displays a contextual menu like a list, which allows the user to select an option from the specified list.
JComboBox can generate an ActionListener interface when the user selects an option. When an option is selected, the method actionPerformed() of ActionListener interface is called and will retrieve the selected value from JComboBox using the method getSelectedItem() of JComboBox class.
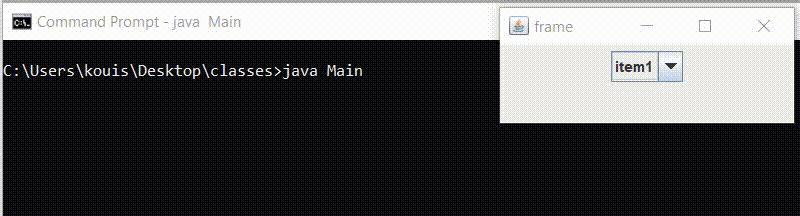
Java Program to get the selected item of a JComboBox:
import javax.swing.*; import java.awt.*; import java.awt.event.*; public class Main extends JFrame { public static void main(String[] argv) { JFrame frame = new JFrame("frame"); frame.setLayout(new FlowLayout()); String[] items = { "item1", "item2", "item3" }; JComboBox cb = new JComboBox(items); cb.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.out.println("Value: " + cb.getSelectedItem().toString()); } }); frame.add(cb); frame.setSize(250, 100); frame.show(); } }
Output:
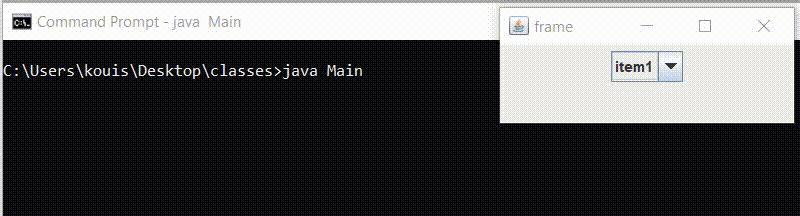