GridBagLayout – Java Swing – Example
In this tutorial, we are going to see an example of GridBagLayout in Java Swing. GridBagLayout is one of the most flexible and complex layout managers provided by the Java platform. GridBagLayout places components in a grid of rows and columns, allowing specified components to span multiple rows or columns. Not all rows are necessarily the same height. Similarly, not all columns are necessarily the same width. Essentially, GridBagLayout places components in rectangles (cells) in a grid, then uses the preferred sizes of the components to determine the size of the cells. The resizing behavior is based on the weights that the program assigns to the components in GridBagLayout.
Example of GridBagLayout in Java Swing
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class MyGridbag extends JFrame { MyGridbag() { // Set the title of JFrame setTitle("GridBagLayout Example"); // Creating an object of Jpanel class JPanel p = new JPanel(); // Set the layout p.setLayout(new GridBagLayout()); // Create a constraint object GridBagConstraints c = new GridBagConstraints(); // Specifies the external padding of all components c.insets = new Insets(1, 1, 1, 1); // column 0 c.gridx = 0; // line 0 c.gridy = 0; // increases the width of the components by 10 pixels c.ipadx = 100; // increases the height of the components by 50 pixels c.ipady = 100; // Add constraints p.add(new JButton("Button 1"), c); // column 1 c.gridx = 1; // increases the width of the components by 90 pixels c.ipadx = 20; // increases the height of the components by 40 pixels c.ipady = 20; // Add constraints p.add(new JButton("Button 2"), c); // column 0 c.gridx = 0; // line 2 c.gridy = 1; // increases the width of the components by 20 pixels c.ipadx = 10; // increases the height of the components by 20 pixels c.ipady = 10; // Add constraints p.add(new JButton("Button 3"), c); // increases the width of the components by 10 pixels c.ipadx = 60; // column 1 c.gridx = 1; // Add constraints p.add(new JButton("Button 4"), c); // Creation of a "WindowAdapter" object WindowListener winAdap = new WindowAdapter() { public void windowClosing(WindowEvent e) { // exit the system System.exit(0); } }; // add the "windowlistener" listener addWindowListener(winAdap); // Add constraints getContentPane().add(p); // Set JFrame size setSize(400, 250); // Set the visibility of JFrame setVisible(true); } // Main method public static void main(String[] args) { // call the constructor new MyGridbag(); } }
Output:
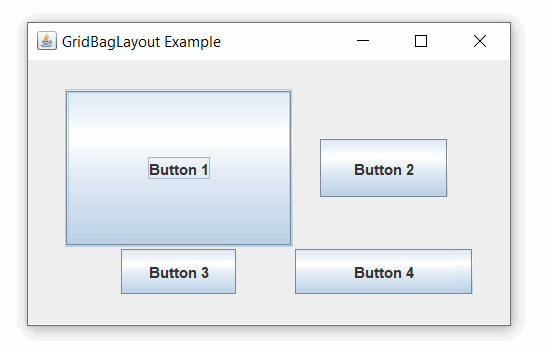