How to link two JComboBox together in Java Swing
In this tutorial, we are going to see how to link two JComboBox together in Java Swing. JComboBox is part of the Java Swing package. JComboBox inherits from JComponent class. JComboBox displays a contextual menu like a list, which allows the user to select an option from the specified list.
JComboBox can generate an ActionListener interface. When an option is selected, actionPerformed() method is called.
In this tutorial, we are going to see how to change data of a JCombobox in relation to another one.
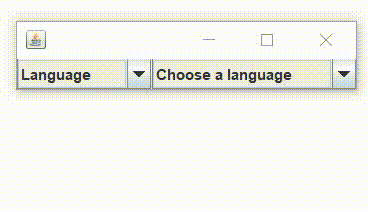
Java Program to link two JComboBox together:
import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.util.*; public class LinkTwoComboBox extends JFrame implements ActionListener { private JComboBox cb1; private JComboBox cb2; private Hashtable hash = new Hashtable(); public LinkTwoComboBox() { String[] items = {"Select an item", "Language", "Color", "Form"}; cb1 = new JComboBox(items); cb1.addActionListener(this); //prevent events from being triggered when the up and down arrow keys are used. cb1.putClientProperty("JComboBox.isTableCellEditor", Boolean.TRUE); getContentPane().add(cb1, BorderLayout.WEST); // create a sublist cb2 = new JComboBox(); cb2.setPrototypeDisplayValue("XXXXXXXXXXXXXXXXX"); getContentPane().add(cb2, BorderLayout.EAST); String[] hash1 = {"Choose a language", "Java", "PHP", "Python"}; hash.put(items[1], hash1); String[] hash2 = {"Choose a color", "Red", "Green", "Blue"}; hash.put(items[2], hash2); String[] hash3 = {"Choose a form", "Triangle", "Circle", "Square"}; hash.put(items[3], hash3); //select the first element by default cb1.setSelectedIndex(1); } public void actionPerformed(ActionEvent e) { String str = (String)cb1.getSelectedItem(); Object o = hash.get(str); if (o == null){ cb2.setModel(new DefaultComboBoxModel()); } else{ cb2.setModel(new DefaultComboBoxModel((String[])o)); } } public static void main(String[] args) { JFrame f = new LinkTwoComboBox(); f.setDefaultCloseOperation(EXIT_ON_CLOSE); f.pack(); f.setLocationRelativeTo(null); f.setVisible(true); } }
Output:
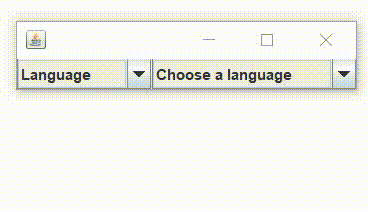