How to Change Look and Feel of Swing Application
In this tutorial, we are going to see how to change the look and feel of the swing application. Java Swing allows us to customize the graphical interface by changing the look and feel (L&F). Look defines the general appearance of the components and Feel defines their behavior. L&Fs are subclasses of LookAndFeel class and each L&F is identified by its full class name. By default, the L&F is set to ‘Metal’.
To set the L&F, we can call the method setLookAndFeel() of the UIManager class. The call to setLookAndFeel must be made before instantiating a Java Swing class, otherwise the default Swing L&F will be loaded.
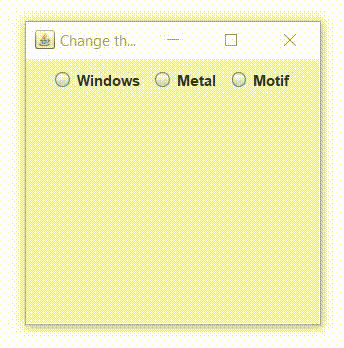
Java Application to Change Look and Feel of Swing Application:
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class TestLF extends JFrame implements ActionListener { private JRadioButton w, ml, mf; private ButtonGroup groupBtn; public TestLF() { setTitle("Change the look of my Swing app"); w = new JRadioButton("Windows"); w.addActionListener(this); ml = new JRadioButton("Metal"); ml.addActionListener(this); mf = new JRadioButton("Motif"); mf.addActionListener(this); groupBtn = new ButtonGroup(); groupBtn.add(w); groupBtn.add(ml); groupBtn.add(mf); setLayout(new FlowLayout()); add(w); add(ml); add(mf); setSize(250, 250); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true); setLocationRelativeTo(null); } @Override public void actionPerformed(ActionEvent actionevent) { String LookAndFeel; if(actionevent.getSource() == w) LookAndFeel = "com.sun.java.swing.plaf.windows.WindowsLookAndFeel"; else if(actionevent.getSource() == mf) LookAndFeel = "com.sun.java.swing.plaf.motif.MotifLookAndFeel"; else LookAndFeel = "javax.swing.plaf.metal.MetalLookAndFeel"; try { UIManager.setLookAndFeel(LookAndFeel); SwingUtilities.updateComponentTreeUI(this); } catch (Exception e) { System.out.println("Error while defining the LookAndFeel..." + e); } } public static void main(String args[]) { new TestLF(); } }
Output:
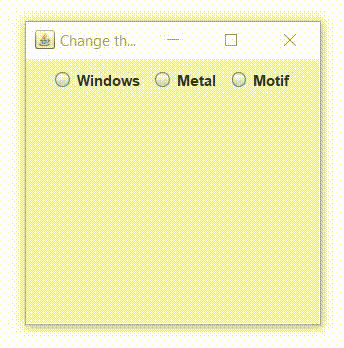