JFrame – Java Swing – Example
In this tutorial, we are going to see an example of JFrame in Java Swing. JFrame is a class found in javax.swing package that inherits from java.awt.frame, it adds support for the SWING component architecture. It is a top-level window, with a border and a title bar. JFrame class has many methods that can be used to customize it.
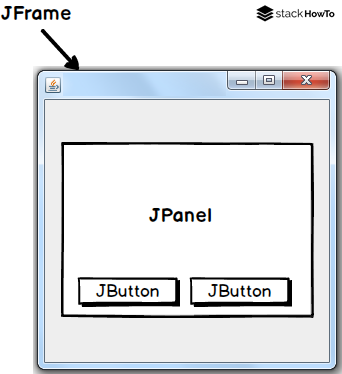
JFrame constructors
JFrame() | Create a frame that is invisible |
JFrame(GraphicsConfiguration gc) | Creates a frame with a blank title and a graphic configuration. |
JFrame(String title) | Create a frame with a title. |
JFrame(String title, GraphicsConfiguration gc) | Create a frame with a specific graphic configuration and a specified title. |
Example : Create a JFrame
Here is a simple example to create a JFrame.
import javax.swing.JFrame; public class Main { public static void main(String[] args) { JFrame frame = new JFrame(); frame.setVisible(true); } }
Output:
Example : Change the size of a JFrame window
To resize a frame, JFrame provides a method JFrame.setSize(int width, int height), it needs two parameters width and height. Here is what the code looks like now:
JFrame frame = new JFrame(); frame.setSize(300, 300); frame.setVisible(true);
Output:
Example: Set the title of JFrame
To set the title of a JFrame, you can use JFrame.setTitle(String title).
JFrame frame = new JFrame(); frame.setSize(300, 300); frame.setTitle("Welecome to StackHowTo!"); frame.setVisible(true);
Output: