How to add onclick event to JButton using ActionListener in Java Swing
In this tutorial, we are going to see how to add onclick event to JButton using ActionListener in Java Swing.
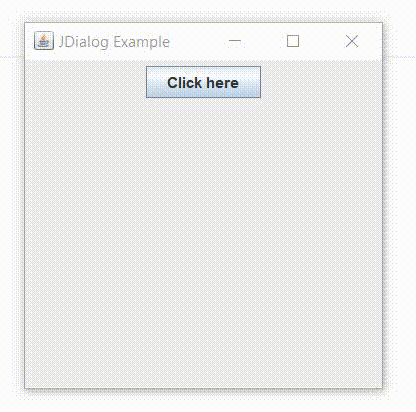
JButton listener
If you want to add onclick event to JButton you need to add an ActionListener to a JButton, as shown in the following code snippet:
JButton btn = new JButton("Button"); btn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { //show jdialog when button is clicked JDialog dialog = new JDialog(frame, "Welcome to StackHowTo", true); dialog.setLocationRelativeTo(frame); dialog.setVisible(true); } });
Place the code in the method “actionPerformed()” of the ActionListener class that you want to execute when the button is clicked. There are several ways to implement this:
- Implement it as shown above
- Request your class to implement an ActionListener
- Create another class as an ActionListener
Complete Example By implementing the ActionListener interface
import java.awt.event.*; import javax.swing.*; import java.awt.*; class JDialogExample extends JFrame implements ActionListener { static JFrame frame; public static void main(String[] args) { // create a frame frame = new JFrame("JDialog Example"); // create an object JDialogExample obj = new JDialogExample(); // create a panel JPanel panel = new JPanel(); JButton btn = new JButton("Click here"); // add a listener to the button btn.addActionListener(obj); // add button to panel panel.add(btn); frame.add(panel); frame.setSize(300, 300); frame.show(); } public void actionPerformed(ActionEvent e) { String s = e.getActionCommand(); if(s.equals("Click here")) { // Create a dialog box JDialog d = new JDialog(frame, "Dialog box"); // Create a label JLabel l = new JLabel("This is a dialog box."); // Add the label to the dialog box d.add(l); // Set the size of the dialog box d.setSize(200, 100); // Define the visibility of the dialog box d.setVisible(true); } } }
Output:
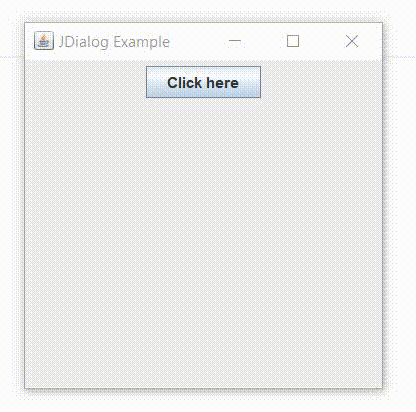