How to Create Multi-Line Header for JTable
In this tutorial, we are going to see how to create multi-line header for JTable. Sometimes, when using JTable to display two-dimensional data, we need to use a long string as a column title. The problem is that the string may not fit in the column because the column title is displayed on a row by default. As a result, the string will be truncated, appended with three dots, like the following table.
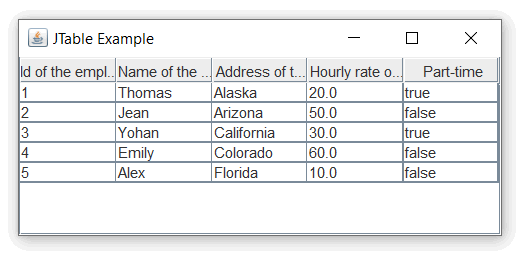
One possible solution is to resize the column to the appropriate size so that the column title is displayed in full. But this will reduce the size of the other columns. Another way is to use the horizontal scroll bar so that users can see the entire table by scrolling horizontally. However, some users may not be happy with this. To display the entire column title without resizing the column or using the horizontal scroll bar, we can make it multiple rows.
We can do this very easily without adding any extra code. Swing allows us to use HTML in several Swing components. To do this, simply add the <html> tag and we can start using various HTML tags. For example, to create a multi-line header , we can use the <br> tag to create a new line:
table.getColumn(1).setHeaderValue("<html><center>Id of<br>the employee</center></html>");
The height of the table header will be automatically increased, so we don’t have to do manually. Here is the result:
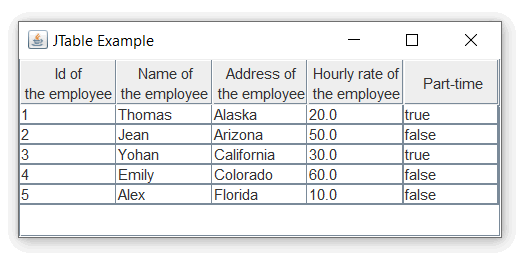
In addition, it is also possible to use other HTML tags to format our text, like this:
table.getColumn(1).setHeaderValue("<html><center><i>Id</i> of<br>the employee</center></html>");
Complete Example :
import javax.swing.*; import java.awt.*; public class Main { public static void main(String[] args) { //create a frame final JFrame frame = new JFrame("JTable Example"); //Headers for JTable String[] columns = new String[] { "<html><center>Id of<br>the employee</center></html>", "<html><center>Name of<br>the employee</center></html>", "<html><center>Address of<br>the employee</center></html>", "<html><center>Hourly rate of<br>the employee</center></html>", "<html><center>Part-time</center></html>" }; //data for JTable in a 2D table Object[][] data = new Object[][] { {1, "Thomas", "Alaska", 20.0, true }, {2, "Jean", "Arizona", 50.0, false }, {3, "Yohan", "California", 30.0, true }, {4, "Emily", "Colorado", 60.0, false }, {5, "Alex", "Florida", 10.0, false }, }; //create a JTable with data JTable table = new JTable(data, columns); JScrollPane scroll = new JScrollPane(table); table.setFillsViewportHeight(true); //add table to frame frame.getContentPane().add(scroll); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(400, 180); frame.setVisible(true); } }
Output:
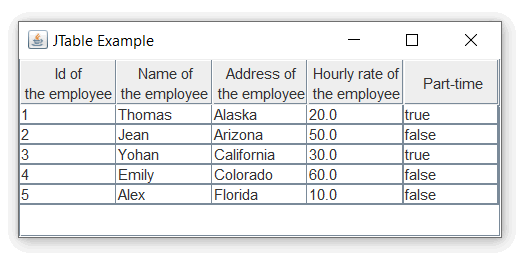