JSlider – Java Swing – Example
In this tutorial, we are going to see an example of JSlider in Java Swing. JSlider is part of Java Swing package. JSlider is an implementation of Slider. The component allows the user to select a value by dragging the button according to the limited value. The slider can display major scales and also minor scales between two major scales. The button can be positioned only at these points.
JSlider constructors class:
JSlider() | Create a new slider with horizontal orientation, max and min values 100 and 0 respectively and the slider value is set to 50 by default. |
JSlider(BoundedRangeModel b) | Creates a new Slider with a specified horizontal orientation and limit range. |
JSlider(int min, int max) | Create a new slider with a horizontal orientation and specified max and min values and the slider value is set to the average of the max and min values. |
JSlider(int min, int max, int value) | Create a new slider with a horizontal orientation, max, min and slider value are specified. |
JSlider(int o, int min, int max, int value) | Create a new slider with an orientation, the max, min and value of the slider are specified. |
Commonly used methods:
- setMaximum(int m): set the maximum value for the slider
- setMinimum(int m): set the minimum value for the slider
- getMinimum(): returns the minimum value
- getMaximum(): returns the maximum value
- setFont(Font f): sets the text font for the slider
- setOrientation(int n): sets the slider orientation to the specified value
- setValue(int n): sets the slider value to the specified value
- setMinorTickSpacing(int n): is used to set the spacing of the minor scales on the slider.
- setMajorTickSpacing(int n): is used to set the spacing of the major scales on the slider.
- setPaintTicks(boolean b): is used to determine if the scales are painted.
- setPaintLabels(boolean b): is used to determine if the labels are painted.
- setPaintTracks(boolean b): is used to determine if the track is painted.
Example 1 of JSlider in Java Swing:
import javax.swing.*; public class SliderTest extends JFrame { public SliderTest() { JSlider s = new JSlider(); JPanel panel = new JPanel(); panel.add(s); add(panel); } public static void main(String s[]) { SliderTest f = new SliderTest(); f.pack(); f.setVisible(true); } }
Output:
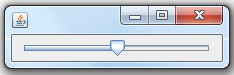
Example 2 of JSlider in Java Swing:
import javax.swing.event.*; import java.awt.*; import javax.swing.*; class SliderTest extends JFrame implements ChangeListener { static JSlider slider; static JLabel label; public static void main(String[] args) { // Create a new frame JFrame frame = new JFrame("Slider Example"); // Create an object SliderTest obj = new SliderTest(); // Create a label label = new JLabel(); // Create a panel JPanel p = new JPanel(); // Create a slider slider = new JSlider(0, 100, 20); // Paint the track and label slider.setPaintTrack(true); slider.setPaintTicks(true); slider.setPaintLabels(true); // Set the spacing slider.setMajorTickSpacing(20); slider.setMinorTickSpacing(5); // Associate the Listener with the slider slider.addChangeListener(obj); // Add the slider to the panel p.add(slider); p.add(label); // Add the panel to the frame frame.add(p); // Set the label text label.setText("The value of the Slider is : " + slider.getValue()); frame.setSize(300, 150); frame.show(); } // Run this method if the value of the slider is modified public void stateChanged(ChangeEvent e) { label.setText("The value of the Slider is : " + slider.getValue()); } }
Output:
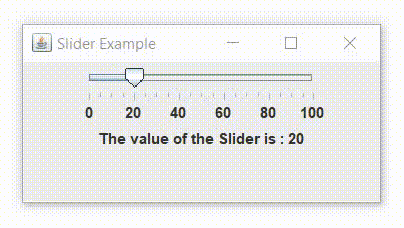