How to Set Column Width in JTable in Java
In this tutorial, we are going to see how to set column width in JTable in Java. When working with JTable in Swing, sometimes we need the table to have columns of different widths, other than the default values provided by the JTable component.
Let’s look at an example from a Swing program, how to change the default value of column width in a JTable:
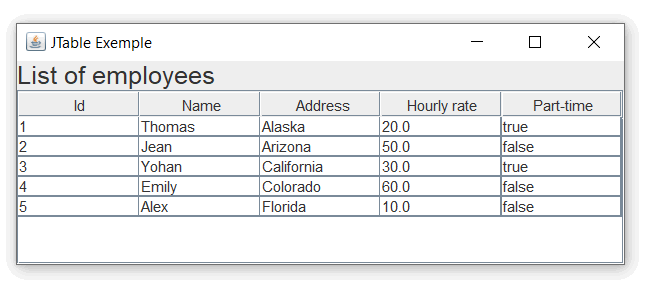
How to Set Column Width in JTable in Java
This can be done easily by using setPreferredWidth() method of TableColumnModel class. For example, the following statement sets the width of column 1 to 20 pixels:
TableColumnModel columnModel = table.getColumnModel(); columnModel.getColumn(0).setPreferredWidth(20);
Output:
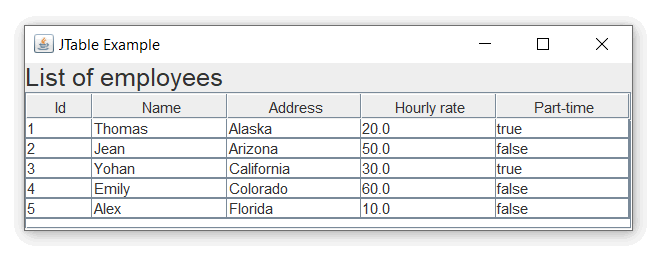
However, setting the preferred width for a single column does not work. The table needs to know the preferred width of all the columns, so the following statements define the preferred width for all 5 columns of the table:
TableColumnModel columnModel = table.getColumnModel(); columnModel.getColumn(0).setPreferredWidth(20); columnModel.getColumn(1).setPreferredWidth(40); columnModel.getColumn(2).setPreferredWidth(40); columnModel.getColumn(3).setPreferredWidth(100); columnModel.getColumn(4).setPreferredWidth(100);
Output:
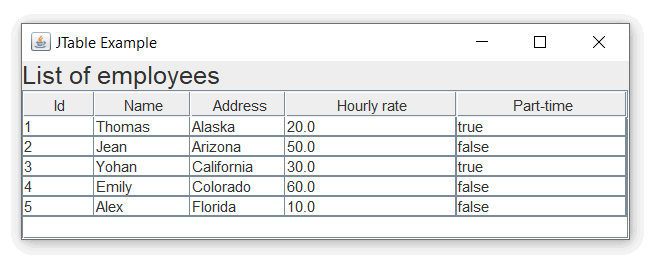
Complete Example :
import javax.swing.*; import java.awt.*; import javax.swing.table.TableColumnModel; public class Main { public static void main(String[] args) { //create a frame final JFrame frame = new JFrame("JTable Example"); //Headers for JTable String[] columns = new String[] { "Id", "Name", "Address", "Hourly rate", "Part-time" }; //data for JTable in a 2D table Object[][] data = new Object[][] { {1, "Thomas", "Alaska", 20.0, true }, {2, "Jean", "Arizona", 50.0, false }, {3, "Yohan", "California", 30.0, true }, {4, "Emily", "Colorado", 60.0, false }, {5, "Alex", "Florida", 10.0, false }, }; //create a JTable with data JTable table = new JTable(data, columns); TableColumnModel columnModel = table.getColumnModel(); columnModel.getColumn(0).setPreferredWidth(20); columnModel.getColumn(1).setPreferredWidth(40); columnModel.getColumn(2).setPreferredWidth(40); columnModel.getColumn(3).setPreferredWidth(100); columnModel.getColumn(4).setPreferredWidth(100); JScrollPane scroll = new JScrollPane(table); table.setFillsViewportHeight(true); JLabel labelHead = new JLabel("List of employees"); labelHead.setFont(new Font("Arial",Font.TRUETYPE_FONT,20)); frame.getContentPane().add(labelHead,BorderLayout.PAGE_START); //add table to frame frame.getContentPane().add(scroll,BorderLayout.CENTER); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(500, 180); frame.setVisible(true); } }
Output:
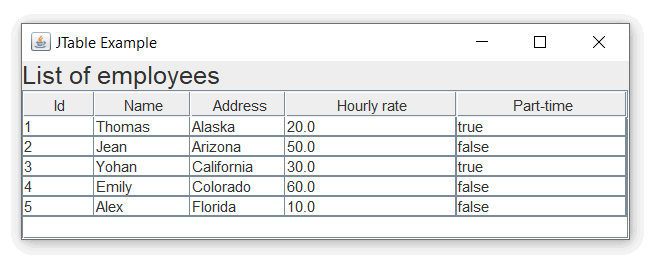