How to Sort JTable Column in Java [2 Methods]
In this tutorial, we are going to see how to sort JTable column in Java. JTable is a flexible Swing component that is very well suited to display data in a tabular format. Sorting rows by columns is a nice feature provided by the JTable class. In this tutorial, we are going to see two methods to sort rows in JTable.
Method 1: Enable sorting for JTable
Use the following statement to enable the table’s default sorting:
table.setAutoCreateRowSorter(true);
Now run the program and click on the Header of the column(the little arrow is not displayed only when you click on the column). We can see that the rows of the table are sorted by that column in ascending order, and vice versa if we click again:
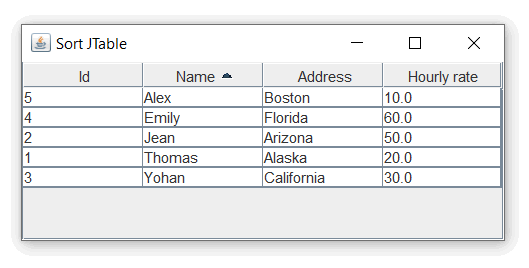
Now the user can click on any header to sort the table data by that column. However, do you notice that the table is not sorted when the program is launched? How to get the table sorted by default? The answer is described in the following method just below.
Method 2: Sort JTable Column Programmatically:
To sort the table by column programmatically, we need to create a TableRowSorter and a SortKey that specifies the column index (by which the table is sorted) and the sort order (ascending or descending). For example, the following code sorts the 2nd column (at index 1) in ascending order:
import java.awt.*; import java.util.*; import javax.swing.*; import javax.swing.table.*; public class SortJTable { public static void main(String[] args) { new SortJTable(); } public SortJTable() { EventQueue.invokeLater(new Runnable() { @Override public void run() { //JTable Header String[] columns = new String[] { "Id", "Name", "Address", "Hourly rate" }; //data for JTable in a 2D table Object[][] data = new Object[][] { {1, "Thomas", "Alaska", 20.0 }, {2, "Jean", "Arizona", 50.0 }, {3, "Yohan", "California", 30.0 }, {4, "Emily", "Florida", 60.0 }, {5, "Alex", "Boston", 10.0 } }; //create a JTable with data JTable table = new JTable(data, columns); TableRowSorters = new TableRowSorter (table.getModel()); //sort JTable rows table.setRowSorter(s); java.util.List sortList = new ArrayList<>(5); sortList.add(new RowSorter.SortKey(1, SortOrder.ASCENDING)); sortList.add(new RowSorter.SortKey(0, SortOrder.ASCENDING)); s.setSortKeys(sortList); JFrame f = new JFrame("Sort JTable"); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.add(new JScrollPane(table)); f.setSize(400, 180); f.setLocationRelativeTo(null); f.setVisible(true); } }); } }
Output:
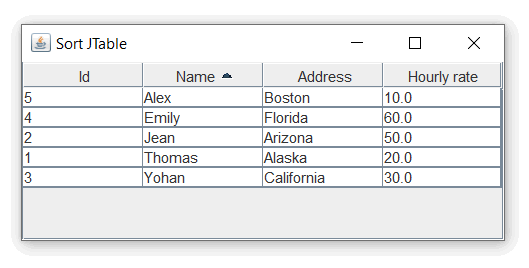