JToggleButton – Java Swing – Example
In this tutorial, we are going to see an example of JToggleButton in Java Swing. JToggleButton is a button with two states (selected and deselected). JRadioButton and JCheckBox classes are subclasses of this class. When the user presses the toggle button, it switches between clicked and unclicked. JToggleButton is used to select a choice from a list of possible choices. Buttons can be configured and controlled by actions. Using an action with a button has many advantages beyond the direct configuration of a button.
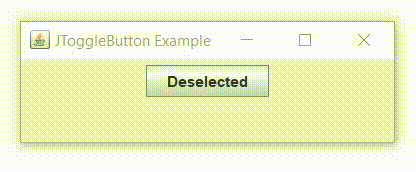
JToggleButton constructors class:
JToggleButton() | Creates an initially unselected ToggleButton without defining the text or image. |
JToggleButton(Action a) | Creates a ToggleButton where the properties are extracted from the provided action. |
JToggleButton(Icon icon) | Creates an initially unselected ToggleButton with the specified image but no text. |
JToggleButton(Icon icon, boolean selected) | Creates a ToggleButton with the specified image and selection state, but no text. |
JToggleButton(String text) | Creates an unselected ToggleButton with the specified text. |
JToggleButton(String text, boolean selected) | Creates a ToggleButton with the specified text and selection state. |
JToggleButton(String text, Icon icon) | Creates a ToggleButton that has the specified text and image, and is initially unselected. |
JToggleButton(String text, Icon icon, boolean selected) | Creates a ToggleButton with the specified text, image and selection state. |
Commonly used methods:
- getAccessibleContext(): Gets the AccessibleContext associated with this JToggleButton.
- getUIClassID(): Returns a string that specifies the name of the Look&Feel class that renders this component.
- paramString(): Returns a string representation of this JToggleButton.
- updateUI(): Resets the user interface to the current appearance.
Example of JToggleButton in Java Swing:
import javax.swing.*; import java.awt.*; import java.awt.event.*; public class MyJToggleButton extends JFrame implements ItemListener { private JToggleButton btn; MyJToggleButton() { setTitle("JToggleButton Example"); setLayout(new FlowLayout()); setJToggleButton(); setAction(); setSize(200, 100); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } private void setJToggleButton() { btn = new JToggleButton("Deselected"); add(btn); } private void setAction() { btn.addItemListener(this); } public void itemStateChanged(ItemEvent eve) { if (btn.isSelected()) btn.setText("Selected"); else btn.setText("Deselected"); } public static void main(String[] args) { new MyJToggleButton(); } }
Output:
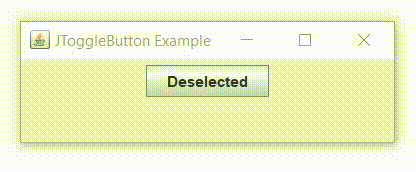