JDialog – Java Swing – Example
In this tutorial, we are going to see an example of JDialog in Java Swing. JDialog is part of the Java swing package. The main purpose of JDialog is to add components to it. JDialog can be customized according to the user’s needs.
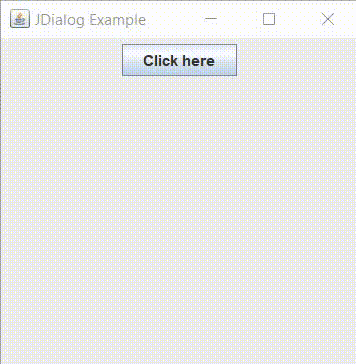
JDialog constructors class:
JDialog() | Creates an empty dialog box without any specified title |
JDialog(Frame o) | Creates an empty dialog box with a specified frame |
JDialog(Frame o, String s) | Creates an empty dialog box with a specified frame and a specified title |
JDialog(Window o) | Creates an empty dialog box with a specified window object |
JDialog(Window o, String t) | Creates an empty dialog box with a window object and a specified title. |
JDialog(Dialog o) | Creates an empty dialog box with a specified dialog box |
JDialog(Dialog o, String s) | Creates an empty dialog box with a specified dialog box and title. |
Commonly used methods:
- setLayout(LayoutManager m) : Sets the layout of the dialog box to the specified layout manager.
- setJMenuBar(JMenuBar m) : Sets the menu bar of the dialog box to the specified menu bar
- add(Component c) : Adds a component to the dialog box
- isVisible(boolean b) : Sets the visibility of the dialog box, if the boolean value is true then visible otherwise invisible
- update(Graphics g) : Call the function paint(g)
- remove(Component c) : Delete the component c
- getJMenuBar() : Returns the menu bar of the component
- remove(Component c) : Removes the specified component from the dialog box.
- setContentPane(Container c) : Sets the content of the dialog box
Example of JDialog in Java Swing:
import java.awt.event.*; import javax.swing.*; import java.awt.*; class JDialogExample extends JFrame implements ActionListener { static JFrame frame; public static void main(String[] args) { // create a frame frame = new JFrame("JDialog Example"); // create an object JDialogExample obj = new JDialogExample(); // create a panel JPanel panel = new JPanel(); JButton btn = new JButton("Click here"); // add listener to button btn.addActionListener(obj); // add button to panel panel.add(btn); frame.add(panel); frame.setSize(300, 300); frame.show(); } public void actionPerformed(ActionEvent e) { String s = e.getActionCommand(); if(s.equals("Click here")) { // Create a dialog JDialog d = new JDialog(frame, "Dialog box"); // Create a label JLabel l = new JLabel("This is a dialog box."); // Add the label to the dialog box d.add(l); // Set the size of the dialog box d.setSize(200, 100); // Set the visibility of the dialog box d.setVisible(true); } } }
Output:
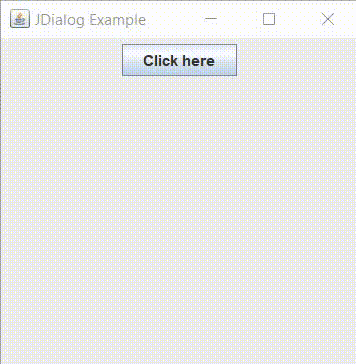