How to Create Hyperlink with JLabel in Java
In this tutorial, we are going to see how to create hyperlink using JLabel component, as Swing does not have any built-in components that can display hyperlinks.
First, create a JLabel like this:
JLabel link = new JLabel("www.stackhowto.com");
Set the color of your text as a standard hyperlink (blue):
link.setForeground(Color.BLUE.darker());
To turn the mouse cursor into “Hand Pointer” icon when the user moves the mouse over the label, set its cursor as follows.
link.setCursor(Cursor.getPredefinedCursor(Cursor.HAND_CURSOR));
And to make the label clickable, add a mouse listener to the JLabel:
lien.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { // the user clicked on the label } @Override public void mouseEntered(MouseEvent e) { // the mouse has entered the label } @Override public void mouseExited(MouseEvent e) { // the mouse left the label } });
We redefine the method mouseClicked() to handle the event in which the user clicks the hyperlink. And in this method, we can use Desktop class to open the associated hyperlink, for example:
@Override public void mouseClicked(MouseEvent e) { try { Desktop.getDesktop().browse(new URI("https://stackhowto.com/")); } catch (URISyntaxException e) { e.printStackTrace(); } }
The operating system will open an appropriate program that associates with the URL, usually a browser if the link is a website URL.
To emphasize the text when the user moves the mouse over the link, we can define the HTML code of the text with the tag <a>. So you can redefine the method mouseEntered() like this:
lien.setText("www.stackhowto.com");
And when the mouse is out, put the text back to the original:
lien.setText("www.stackhowto.com");
Complete Example :
import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.io.*; import java.net.*; public class Link extends JFrame { private String str = "www.stackhowto.com"; private JLabel link = new JLabel(str); public Link() throws HeadlessException { super(); setTitle("www.stackhowto.com"); link.setForeground(Color.BLUE.darker()); link.setCursor(new Cursor(Cursor.HAND_CURSOR)); link.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { try { Desktop.getDesktop().browse(new URI("https://stackhowto.com/")); } catch (IOException | URISyntaxException e1) { e1.printStackTrace(); } } @Override public void mouseExited(MouseEvent e) { link.setText(str); } @Override public void mouseEntered(MouseEvent e) { link.setText(str); } }); setLayout(new FlowLayout()); getContentPane().add(link); setSize(300, 200); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new Link().setVisible(true);; } }); } }
Output:
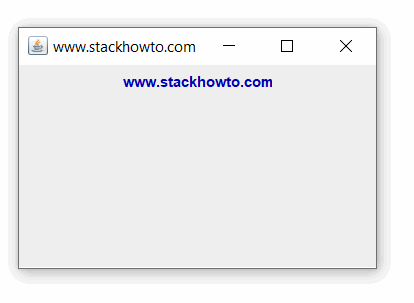