How to Add Image Icon to JButton in Java Swing
In this tutorial, we are going to see how to add image Icon to JButton in Java Swing.
To add an icon to a button, use the class Icon, which will allow you to add an image to a button. In the example below, we create a button in which we add an icon with the class Icon.
Icon icon = new ImageIcon("C:\\image.jpg"); JButton btn = new JButton(icon);
In the above code, we have defined the icon for the btn button.
Example 1: Add Image Icon to JButton
import javax.swing.*; public class ButtonImg { ButtonImg() { JFrame f = new JFrame("Add Image Icon to JButton"); Icon icon = new ImageIcon("subscribe.png"); JButton btn = new JButton(icon); btn.setBounds(40,80,200,50); f.add(btn); f.setSize(300,250); f.setLayout(null); f.setVisible(true); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { new ButtonImg(); } }
Output:
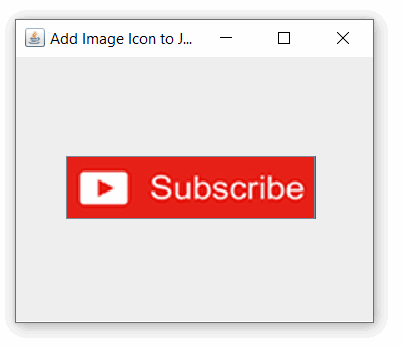
Example 2: Add Image/Text to JButton
import javax.swing.*; public class ButtonImg { ButtonImg() { JFrame f = new JFrame("Add Image/Text to JButton"); Icon icon = new ImageIcon("C:\\setting.png"); // JButton with image / text JButton btn = new JButton("Setting", icon); // Text below the image btn.setVerticalTextPosition(SwingConstants.BOTTOM); // Centered Text btn.setHorizontalTextPosition(SwingConstants.CENTER); btn.setBounds(100,50,80,100); f.add(btn); f.setSize(300,250); f.setLayout(null); f.setVisible(true); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { new ButtonImg(); } }
Output:
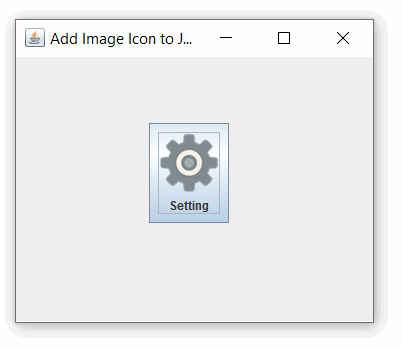