How to Change JButton Text on Click
In this tutorial, we are going to see how to change JButton text on click. JButton is a subclass of AbstractButton class and it can be used to add platform-independent buttons to a Java Swing application. JButton can generate an ActionListener interface when the user clicks on a button, it can also generate MouseListener and KeyListener interfaces.
By default, we can create a JButton with a text and also change the text of a JButton, it will call the actionPerformed() method of the ActionListener interface and set an updated text in a button by calling setText(‘New text’) method of JButton class.
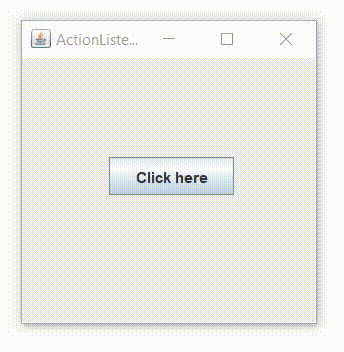
Java Program to Change JButton Text on Click:
import javax.swing.*; import java.awt.event.*; public class JButtonExample { public static void main(String[] args) { JFrame frame = new JFrame("ActionListener Example"); JButton btn = new JButton("Click here"); btn.setBounds(70,80,100,30); //Change button text on click btn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent ae) { btn.setText("OK"); } }); frame.add(btn); frame.setSize(250,250); frame.setLayout(null); frame.setVisible(true); } }
Output:
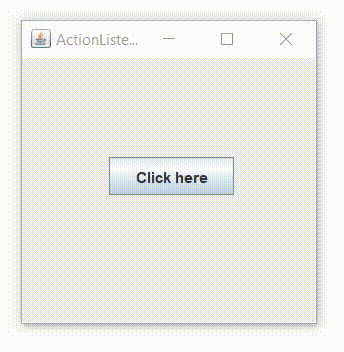