How to Display Multiple Images in a JFrame
In this tutorial, we are going to see how to display multiple images in a JFrame. Graphics class provides the Graphics.drawImage(Image, int x, int y, ImageOberver observer) method to draw an image. Although Image is a class, you can use getDafaultKit() method to get the address of the image. Place your image in ‘images’ folder of your project.
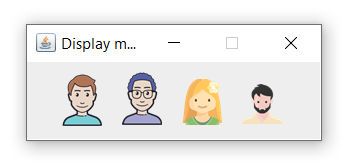
Java Program to Display Multiple Images in a JFrame:
import java.awt.*; import javax.swing.*; public class DrawMyImgs extends JPanel { public void paint(Graphics g){ Image img1 = Toolkit.getDefaultToolkit().getImage("img/user1.png"); g.drawImage(img1, 10, 10, this); Image img2 = Toolkit.getDefaultToolkit().getImage("img/user2.png"); g.drawImage(img2, 70, 8, this); Image img3 = Toolkit.getDefaultToolkit().getImage("img/user4.png"); g.drawImage(img3, 130, 15, this); Image img4 = Toolkit.getDefaultToolkit().getImage("img/user3.png"); g.drawImage(img4, 190, 20, this); } public static void main(String[] args){ JFrame f = new JFrame("Display multiple images"); f.getContentPane().add(new DrawMyImgs()); f.setSize(250, 100); f.setVisible(true); f.setResizable(false); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } }
Output:
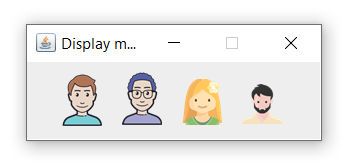