How to Remove Jtable Header in Java
In this tutorial, we are going to see how to remove Jtable header in Java, using setTableHeader() method. Here is an example of our JTable with the header (ID, Name, Address, Hourly rate, Part-time).
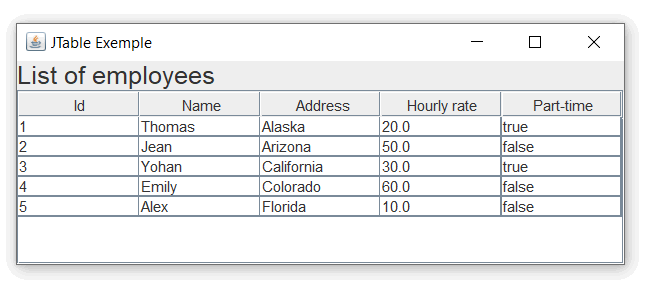
To hide the header of a JTable use setTableHeader() method and set it to null:
JTable table = new JTable(data, columns); table.setTableHeader(null);
Output:
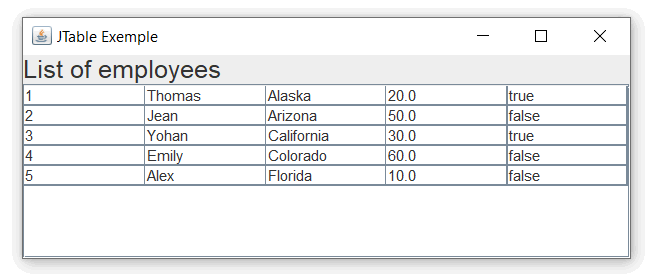
How to Remove Jtable Header in Java
import javax.swing.*; import java.awt.*; public class Main { public static void main(String[] args) { //create a frame final JFrame frame = new JFrame("JTable Example"); //Headers for JTable String[] columns = new String[] { "Id", "Name", "Address", "Hourly rate", "Part-time" }; //data for JTable in a 2D table Object[][] data = new Object[][] { {1, "Thomas", "Alaska", 20.0, true }, {2, "Jean", "Arizona", 50.0, false }, {3, "Yohan", "California", 30.0, true }, {4, "Emily", "Colorado", 60.0, false }, {5, "Alex", "Florida", 10.0, false }, }; //create a JTable with data JTable table = new JTable(data, columns); //set header to null table.setTableHeader(null); JScrollPane scroll = new JScrollPane(table); table.setFillsViewportHeight(true); JLabel labelHead = new JLabel("List of employees"); labelHead.setFont(new Font("Arial",Font.TRUETYPE_FONT,20)); frame.getContentPane().add(labelHead,BorderLayout.PAGE_START); //add table to frame frame.getContentPane().add(scroll,BorderLayout.CENTER); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(500, 200); frame.setVisible(true); } }
Output:
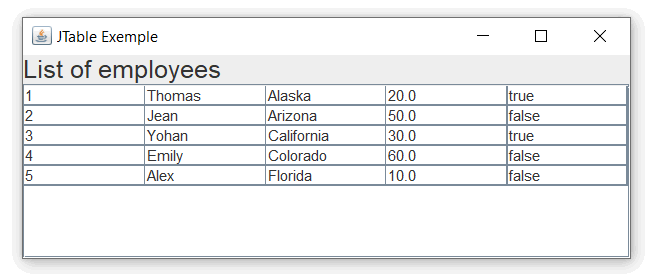