How to add JCheckBox in JTable
In this tutorial, we are going to see how to add JCheckBox in JTable. JTable is a subclass of JComponent class and it can be used to create a table with information displayed in multiple rows and columns. When a value is selected in a JTable, a TableModelEvent is generated, which is handled by implementing a TableModelListener interface. We can add or insert a checkbox in a JTable cell by implementing the method getColumnClass().
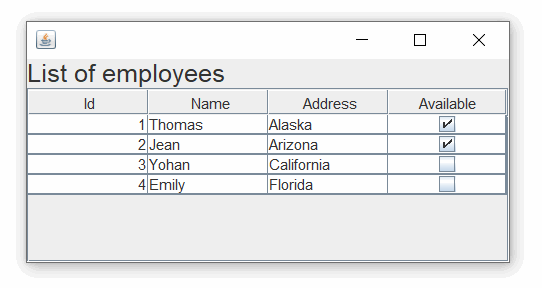
We need to redefine the method getColumnClass() to return Boolean.class for the appropriate column.
Java Program to add JCheckBox in JTable:
import javax.swing.*; import java.awt.*; import javax.swing.table.DefaultTableModel; public class CheckBoxInTable extends JFrame { public CheckBoxInTable() { //Headers for JTable String[] columns = {"Id", "Name", "Address", "Available"}; //data for JTable in a 2D table Object[][] data = { {1, "Thomas", "Alaska", true }, {2, "Jean", "Arizona", true }, {3, "Yohan", "California", false }, {4, "Emily", "Florida", false } }; DefaultTableModel model = new DefaultTableModel(data, columns); JTable table = new JTable(model) { public Class getColumnClass(int column) { //return Boolean.class return getValueAt(0, column).getClass(); } }; JScrollPane scrollPane = new JScrollPane(table); getContentPane().add(scrollPane); JLabel labelHead = new JLabel("List of employees"); labelHead.setFont(new Font("Arial",Font.TRUETYPE_FONT,20)); getContentPane().add(labelHead,BorderLayout.PAGE_START); } public static void main(String[] args) { CheckBoxInTable frame = new CheckBoxInTable(); frame.setDefaultCloseOperation(EXIT_ON_CLOSE); frame.setSize(400, 200); frame.setVisible(true); } }
Output:
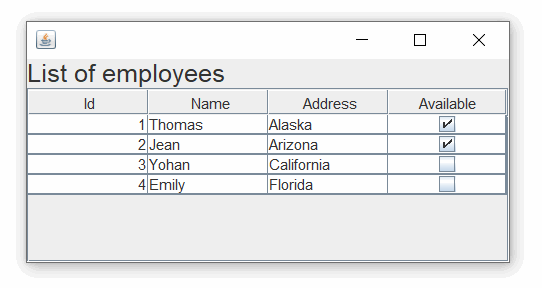