How to Populate JTable from Database
In this tutorial, we are going to see how to populate JTable from a database in Java Swing. The first thing to do is to set up our database.
Create a database called “test”. In the “test” database, add a table called “user”. The user table will take the following three fields.
- id
- name
- age
You can create this using a MySQL client like PHPMyAdmin.
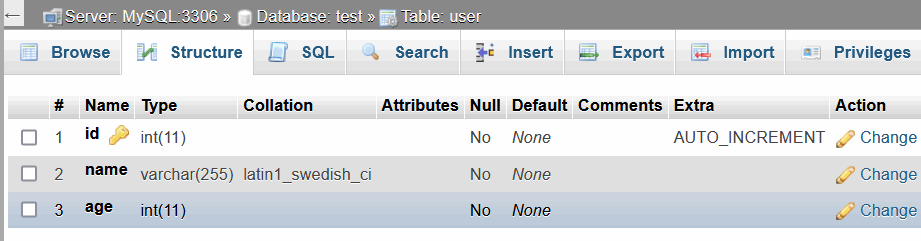
Or you can create it on MySQL command prompt using the following SQL script:
CREATE TABLE `users` ( `id` int(11) NOT NULL AUTO_INCREMENT PRIMARY KEY, `name` varchar(100) NOT NULL, `age` int(2) NOT NULL, ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
To create a table in Java Swing, we will use JTable. javax.swing.JTable class is used to create an instance of a table in Java. javax.swing.table.DefaultTableModel provides the table model. To get better table visibility, it is recommended to put the table in JScrollPane.
You can define the table data in different ways, but the easiest way is to use an array. See the following example.
Java Program to Populate JTable from Database:
import java.sql.*; import javax.swing.*; import javax.swing.table.DefaultTableModel; public class Main { public static void main(String[] args) { try { String url = "jdbc:mysql://localhost:3306/test?autoReconnect=true&useSSL=false"; String user = "root"; String password = ""; Connection con = DriverManager.getConnection(url, user, password); String query = "SELECT * FROM user"; Statement stm = con.createStatement(); ResultSet res = stm.executeQuery(query); String columns[] = { "ID", "Name", "Age" }; String data[][] = new String[8][3]; int i = 0; while (res.next()) { int id = res.getInt("ID"); String nom = res.getString("name"); String age = res.getString("age"); data[i][0] = id + ""; data[i][1] = nom; data[i][2] = age; i++; } DefaultTableModel model = new DefaultTableModel(data, columns); JTable table = new JTable(model); table.setShowGrid(true); table.setShowVerticalLines(true); JScrollPane pane = new JScrollPane(table); JFrame f = new JFrame("Populate JTable from Database"); JPanel panel = new JPanel(); panel.add(pane); f.add(panel); f.setSize(500, 250); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } catch(SQLException e) { e.printStackTrace(); } } }
Output:
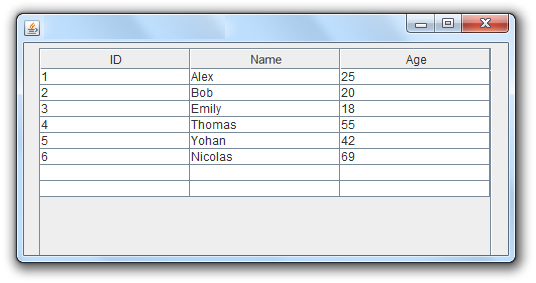
You can execute the above code in command line (CMD):
javac Main.java java -cp .;lib/mysql-connector-java-5.1.49.jar Main
Download mysql-connector-java-5.1.49.jar file and put it in the lib folder of your project.