JCheckBox – Java Swing – Example
In this tutorial, we are going to see an example of JCheckBox in Java Swing. JCheckBox is a Swing component that represents an element that shows a selected or unselected state. The user can change this state by clicking on the checkbox.
A standard JCheckBox component contains a checkbox and a label that describes the purpose of the checkbox. An icon and a mnemonic key can also be defined for this component.
JCheckBox constructors class:
JCheckBox() | Creates a new checkbox without text or icon. |
JCheckBox(Icon i) | Creates a new checkbox with an icon |
JCheckBox(Icon icon, boolean s) | Creates a new checkbox with an icon and the boolean value specifies whether it is selected or not. |
JCheckBox(String t) | Creates a new checkbox with a string |
JCheckBox(String text, boolean selected) | Creates a new checkbox with a string and the boolean value specifies whether it is selected or not. |
JCheckBox(String text, Icon icon) | Creates a new checkbox with the specified string and icon. |
JCheckBox(String text, Icon icon, boolean selected) | Creates a new checkbox with the specified string and icon and the boolean value specifies whether it is selected or not. |
Commonly used methods:
- setIcon(Icon i): sets the checkbox icon to the given icon
- setText(String s): sets the checkbox text to the given text
- setSelected(boolean b): sets the checkbox if the transmitted boolean value is true or vice versa
- getIcon(): returns the image of the checkbox
- getText(): returns the text of the checkbox
- updateUI(): resets the GUI property to the current Look & Feel value.
- getUI(): returns the Look & Feel object which makes this component.
- paramString(): returns a string representation of this JCheckBox.
- getUIClassID(): gets the AccessibleContext associated with this JCheckBox.
- getAccessibleContext(): gets the AccessibleContext associated with this JCheckBox.
- isBorderPaintedFlat(): gets the value of the borderPaintedFlat property.
- setBorderPaintedFlat(boolean b): sets the borderPaintedFlat property.
Example of JCheckBox in Java Swing:
import java.awt.*; import javax.swing.*; class Main extends JFrame { static JFrame f; public static void main(String[] args) { // create a new frame f = new JFrame("Checkbox Example"); // set the frame layout f.setLayout(new FlowLayout()); // create a checkbox JCheckBox check1 = new JCheckBox("Male"); JCheckBox check2 = new JCheckBox("Female"); // create a new panel JPanel p = new JPanel(); // add a checkbox to the panel p.add(check1); p.add(check2); // add panel to frame f.add(p); // set the frame size f.setSize(250, 250); f.show(); } }
Output:
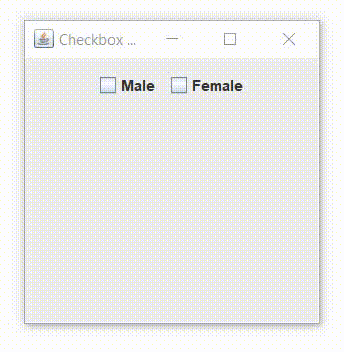