How to Create Rounded JButton in Java
In this tutorial, we are going to see how to create rounded JButton in Java. JButton is a subclass of AbstractButton class and it can be used to add platform-independent buttons in a Java Swing application. In this tutorial, we are going to see how to create a rounded JButton in Java, by implementing Border interface provided under javax.swing.border.Border package.
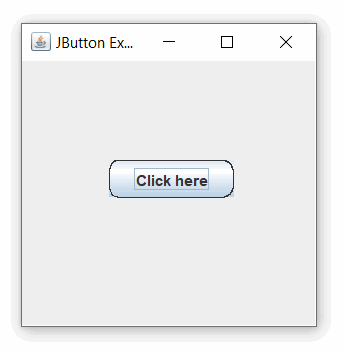
Java Program to Create Rounded JButton:
import javax.swing.*; import java.awt.*; import javax.swing.border.Border; public class Main { public static void main(String[] args) { //create a frame JFrame frame = new JFrame("JButton Example"); //create button JButton btn = new JButton("Click here"); //set button position btn.setBounds(70,80,100,30); //Round the button with radius = 15 btn.setBorder(new RoundBtn(15)); //add button to frame frame.add(btn); frame.setSize(250,250); frame.setLayout(null); frame.setVisible(true); } } class RoundBtn implements Border { private int r; RoundBtn(int r) { this.r = r; } public Insets getBorderInsets(Component c) { return new Insets(this.r+1, this.r+1, this.r+2, this.r); } public boolean isBorderOpaque() { return true; } public void paintBorder(Component c, Graphics g, int x, int y, int width, int height) { g.drawRoundRect(x, y, width-1, height-1, r, r); } }
Output:
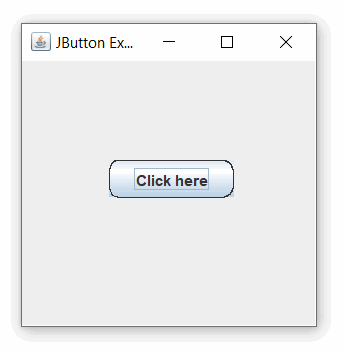
this isnt even round…
You can change the value of radius to make it rounded. In the above example radius = 15.
Change the parameter of RoundBtn function to make the Jbutton rounded.
How can I add color to this ?
Is it possible to have one color inside the border and another color outside of it?