Insertion Sort in Python
admin
0 Comments
algorithm, insertion sort, insertion sort in python, insertion sort in python using for loop, insertion sort in python with example, sorting algorithm
We can create a Python script to sort the array elements using insertion sort. The insertion sort algorithm is only useful for small elements, as it takes more time to sort a large number of elements. Here is how the process works:

Source: Wikipedia.org
Insertion Sort in Python
# Python script for implementing Insertion Sort def insertion_sort(arr): # Loop from 1 to arr size for i in range(1, len(arr)): k = arr[i] j = i-1 while j >= 0 and k < arr[j] : arr[j + 1] = arr[j] j -= 1 arr[j + 1] = k # Main program to test the above code arr = [98, 22, 15, 32, 2, 74, 63, 70] insertion_sort(arr) print ("Sorted array:") for i in range(len(arr)): print ("% d" % arr[i])
Output:
Sorted array: 2 15 22 32 63 70 74 98
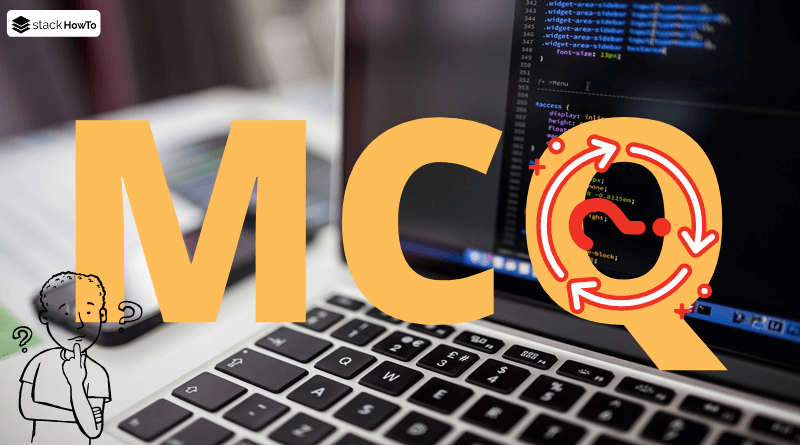
- Button Tkinter | Python 3
- Label Tkinter | Python 3
- Text Tkinter | Python 3
- Entry Tkinter | Python 3
- Message Tkinter | Python 3
- Checkbutton Tkinter | Python 3
- Radiobutton Tkinter | Python 3
- Menu Tkinter | Python 3
- Menubutton Tkinter | Python 3
- Frame Tkinter | Python 3
- Scrollbar Tkinter | Python 3
- Spinbox Tkinter | Python 3
- Listbox Tkinter | Python 3
- Canvas Tkinter | Python 3
- tkMessageBox Tkinter | Python 3
- LabelFrame Tkinter | Python 3
- Scale Tkinter | Python 3
- Toplevel Tkinter | Python 3
- PanedWindow Tkinter | Python 3
- pack() Method in Tkinter Python 3
- grid() Method in Tkinter Python 3
- place() Method in Tkinter Python 3
- How to Get Window Size in Tkinter Python
- How to Set Default Tkinter Entry Value in Python
- How to Change the Default Icon on a Tkinter Window
- How to Bind The Enter key to a Function in Tkinter
- How to Clear Text From a Text Widget on Click in Tkinter
- How to Set The Width and Height of a Tkinter Entry
- How to Get the Input From the Tkinter Text Widget
- How to Make Tkinter Text Widget Read Only
- How to Increase Font Size in Text Widget in Tkinter
- How to Get the Tkinter Label Text
- How To Show/Hide a Label in Tkinter After Pressing a Button
- How to Delete Tkinter Button After Click
- How to Change Label Text on Button Click in Tkinter
- How to Change the Font Size in a Label in Tkinter Python
- How to Make a Timer in Python Tkinter
- How To Display a Tkinter Window in Fullscreen
- How to Stop a Tkinter Window From Resizing
- How to Open a New Window With a Button in Python Tkinter
- How to Disable/Enable Tkinter Button in Python
- How to Close a Tkinter Window With a Button
- How to Pass Arguments to Tkinter button’s callback Command?
- How to Bind Multiple Commands to Tkinter Button
- How to Change Background Color of the Window in Tkinter Python
- How to Change Background Color of a Tkinter Button in Python
- How to Change Text of Button When Clicked in Tkinter Python
- How to change font and size of buttons in Tkinter Python
- How to Set Tkinter Window Size – Python
- How To Add Image To Button In Python Tkinter
- How to run a python script from the command line in Windows 10
- How to install Python on Windows
- How to Install Pip for Python on Windows
- Python Program to Check a Number or String is Palindrome
- Palindrome Program In Python Using Recursion
- What is Django used for?
- Python – Generate a Random Alphanumeric String
- How to generate a random number in Python
- Python – Check if Variable is a Number
- Python Program to Check Armstrong Number
- Python Program to Check if a Year is a Leap Year
- Python program to convert decimal to binary
- Check if a number is odd or even in Python
- Factorial in Python
- Factorial of a Number Using Recursion in Python
- Selection Sort in Python
- Insertion Sort in Python
- Bubble Sort in Python
- How to check if a list is empty in Python
- How to Count Occurrences of Each Character in String – Python
- Python – Read a file line-by-line
- How to get the path of the current directory in Python
- How to get file creation and modification date in Python
- How to extract a zip file in Python
- How to Recursively Remove a Directory in Python
- How to check if a file or a directory exists in Python
- How to move a file or directory in Python
- How to create a directory in Python
- How to list all the files in a directory in Python
- How to delete a file or directory in Python
- How to check if a directory is empty in Python
- Python – How to copy files from one directory to another
- How to Create Nested Dictionary in Python
- How to add keys and values to a dictionary in Python
- How to copy a dictionary in Python
- How to get key by value in dictionary in Python
- Python – Check if String Contains Substring
- Python – Remove duplicates from a list
- How to Remove More Than One Element From List in Python
- Python – Convert a Tuple to a String
- Python – Convert list of tuples to list of lists
- Python – Convert a list of tuples into list
- How to Convert a List into a Tuple in Python
- How to Convert a String to Float in Python
- How to Convert a String to an integer in Python
- How To Convert String To List in Python
- How to Convert List to String in Python
- How to Sort a Dictionary by Value in Python
- How to Sort a Dictionary by Key in Python
- How to Check if an Item Exists in a List in Python
- Python – Check if all elements in a List are the same
- How to merge two lists in Python
- Python – How to Insert an element at a specific index in List
- Python Check If a List Contains Elements of Another List
- Write a Program to Print the Sum of Two Numbers in Python
- How to convert a list of key-value pairs to dictionary Python
- Fibonacci Series in Python using Recursion
- Fibonacci Series in Python using While Loop
- Python Program to Display Prime Numbers in a Given Range
- Python MCQ and Answers – Part 1
- Python MCQ and Answers – Part 2
- Python MCQ and Answers – Part 3
- Python MCQ and Answers – Part 4
- Python MCQ and Answers – Part 5
- Python MCQ and Answers – Part 6
- Python MCQ and Answers – Part 7
- Python MCQ and Answers – Part 8
- Python MCQ and Answers – Part 9
- Python MCQ and Answers – Part 10
- Python MCQ and Answers – Part 11
- Python MCQ and Answers – Part 12
- Python MCQ and Answers – Part 13
- Python MCQ and Answers – Part 14
- Python MCQ and Answers – Part 15
- Python MCQ and Answers – Part 16
- Python MCQ and Answers – Part 17
- Python MCQ and Answers – Part 18
- Python MCQ and Answers – Part 19
- Python MCQ and Answers – Part 20
- Lists in Python
- String Functions and Methods in Python
- Using Variables In Python
- Output using print() function in Python
- Python MCQ and Answers – Lists
- Python MCQ and Answers – Strings
- Python MCQ and Answers – Data types
- Python MCQ and Answers – Variables And Operators – Part 2
- Python MCQ and Answers – Variables And Operators – Part 1