How to Create Nested Dictionary in Python
In this tutorial, we are going to see how to create nested dictionary in Python. A dictionary in Python works in a similar way to a dictionary in the real world. The keys of a dictionary must be unique and have an immutable data type, such as string, integer, etc… but the values can be repeated many times and be of any type.
In Python, a dictionary is an unordered collection. For example:
dictionary = {'key1' : 'value1', 'key2': 'value2'}
What is a nested dictionary in Python?
In Python, a nested dictionary is a dictionary within another dictionary.
nested_dictionary = { 'dict_1': { 'key_1': 'value_1' }, 'dict_2': { 'key_2': 'value_2' } }
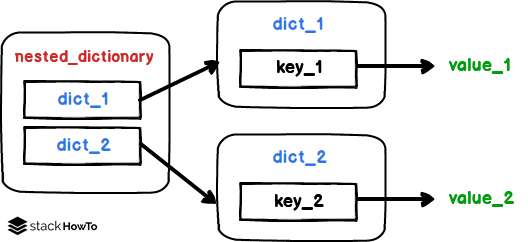
How to Create Nested Dictionary in Python
In the code below, “students” is a dictionary. Nested dictionaries 1 and 2 are assigned to the “students” dictionary. Here, the two nested dictionaries have the keys “name” and “age” with different values. Next, we display the “students” dictionary.
students = { 1: { 'name': 'Alex', 'age': '15' }, 2: { 'name': 'Bob', 'age': '29' } } print(students)
Output:
{1: {'name': 'Alex', 'age': '15'}, 2: {'name': 'Bob', 'age': '29'}}
Access the elements of a nested dictionary
students = { 1: { 'name': 'Alex', 'age': '15' }, 2: { 'name': 'Bob', 'age': '29' } } print(students[1]['name']) print(students[1]['age'])
Output:
Alex 15
Add an element to a nested dictionary
students = { 1: { 'name': 'Alex', 'age': '15' }, 2: { 'name': 'Bob', 'age': '29' } } students[3] = {} students[3]['name'] = 'Jean' students[3]['age'] = '22' print(students[3])
Output:
{'name': 'Jean', 'age': '22'}