Listbox Tkinter | Python 3
In this tutorial, we are going to see how to use Listbox in Tkinter. The Listbox widget allows to show a list of elements from which a user can select a number of elements.
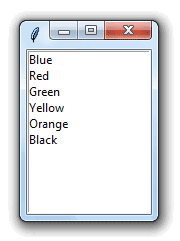
Syntax:
Here is the syntax to create this widget:
liste = Listbox ( master, option = value, ... )
Parameters:
- master : This represents the parent window.
- options : See below for a list of the most commonly used options for this widget. These options can be used as comma separated key-value pairs.
Example:
from tkinter import * gui = Tk() list = Listbox(gui) list.insert(1, "Blue") list.insert(2, "Red") list.insert(3, "Green") list.insert(4, "Yellow") list.insert(5, "Orange") list.insert(6, "Black") liste.pack() gui.mainloop()
Output:
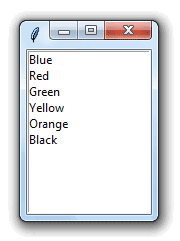
Listbox widget options
bd | Border width in pixels. The default value is 2. |
bg | Background color. |
fg | Foreground color (text). |
font | Text font to be used for the list. |
height | Number of lines (not pixels!) displayed in the list box. The default value is 10. |
highlightcolor | The color of the focus when the widget has the focus. |
selectmode | Defines the number of items that can be selected and how the items are selected:
|
relief | Relief indicates the type of border. Some of the values are SUNKEN, RAISED, GROOVE and RIDGE. |
width | The width of the widget in characters. The default value is 20. |
xscrollcommand | If you want to allow the user to scroll horizontally, you can link your listbox widget to a horizontal scroll bar. |
yscrollcommand | If you want to allow the user to scroll vertically, you can link your listbox widget to a vertical scroll bar. |
Methods:
Here are the commonly used methods for this widget:
activate(index) | Selects the row specified by the given index. |
curselection() | Returns a tuple containing the row numbers of the selected item(s), counting from 0. If nothing is selected, returns an empty tuple. |
delete(first, last=None) | Deletes the rows whose indices are in the range [first, last]. If the second argument is omitted, the row with index first is deleted. |
get(first, last=None) | Returns a tuple containing the text of rows whose indices are in the range [first, last], last is included. If the second argument is omitted, returns the text of the row closest to the first. |
index(i) | Set the visible part of the list so that the row containing the index i is at the top of the widget. |
insert(index, *elements) | Insert one or more new rows in the list before the row specified by index. Use END as the first argument if you want to add new rows at the end of the list. |
nearest(y) | Returns the index of the visible row closest to the y coordinate. |
see(index) | Adjust the position of the list so that the row referenced by the index is visible. |
size() | Returns the number of rows in the list. |