How to Change Label Text on Button Click in Tkinter
In this tutorial, we are going to see different ways to change label text on button click in Tkinter Python.
- Method 1: Using StringVar constructor
- Method 2: Using ‘text’ property of the label widget
Change Label Text Using StringVar
StringVar is a type of Tkinter constructor to create a variable of type String. After binding the StringVar variable to the Tkinter Label widgets, Tkinter will update this widget when the variable is modified.
import tkinter as tk def changeText(): text.set("Welcome to StackHowTo!") gui = tk.Tk() gui.geometry('300x100') text = tk.StringVar() text.set("Hello World!") label = tk.Label(gui, textvariable=text) label.pack(pady=20) button = tk.Button(gui, text="Change the text", command=changeText) button.pack() gui.mainloop()
Output:
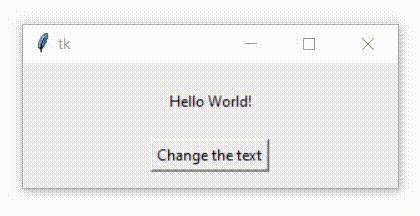
Change Label Text Using ‘text’ Property
Another way to change the text of the Tkinter label is to change the ‘text’ property of the label.
import tkinter as tk def changeText(): label['text'] = "Welcome to StackHowTo!" gui = tk.Tk() gui.geometry('300x100') label = tk.Label(gui, text="Hello World!") label.pack(pady=20) button = tk.Button(gui, text="Change the text", command=changeText) button.pack() gui.mainloop()
Output:
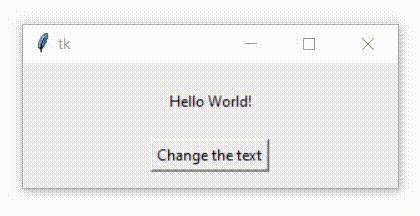
We can also change the ‘text’ property with tk.Label.configure() method as shown below. It works the same way with the codes above.
import tkinter as tk def changeText(): label.configure(text="Welcome to StackHowTo!") gui = tk.Tk() gui.geometry('300x100') label = tk.Label(gui, text="Hello World!") label.pack(pady=20) button = tk.Button(gui, text="Change the text", command=changeText) button.pack() gui.mainloop()